Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |
Tags
- Clean Code
- swiftUI
- map
- tableView
- Refactoring
- uiscrollview
- 클린 코드
- scrollview
- ios
- RxCocoa
- uitableview
- collectionview
- 스위프트
- 리팩토링
- clean architecture
- ribs
- Observable
- SWIFT
- UICollectionView
- HIG
- Protocol
- Xcode
- swift documentation
- Human interface guide
- UITextView
- 애니메이션
- rxswift
- combine
- MVVM
- 리펙토링
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] UITableView의 grouped 스타일 사용시 주의 사항 (footer 영역 제거 방법) 본문
iOS 응용 (swift)
[iOS - swift] UITableView의 grouped 스타일 사용시 주의 사항 (footer 영역 제거 방법)
jake-kim 2023. 2. 8. 22:42UITableView의 대표적인 2가지 style
- header, footer가 없으면 둘 차이 x
- plain 스타일 - 스크롤 시 header가 상단에 고정 (sticky header 처럼 보여지는 효과)
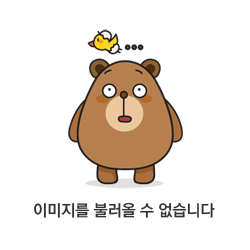
- grouped 스타일 - 스크롤 시 header가 상단에 고정되지 않고, header가 있으면 자동으로 footer 영역이 생성
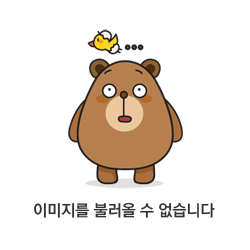
grouped 스타일 주의사항
- 보통 section이 고정되지 않게끔 구현해야하기 때문에 grouped 스타일을 많이 사용
- grouped 스타일을 사용하고 header를 주면 개발자 의도에 상관없이 footer 영역이 자동으로 생성되므로 footer를 없애주는 코드가 따로 필요
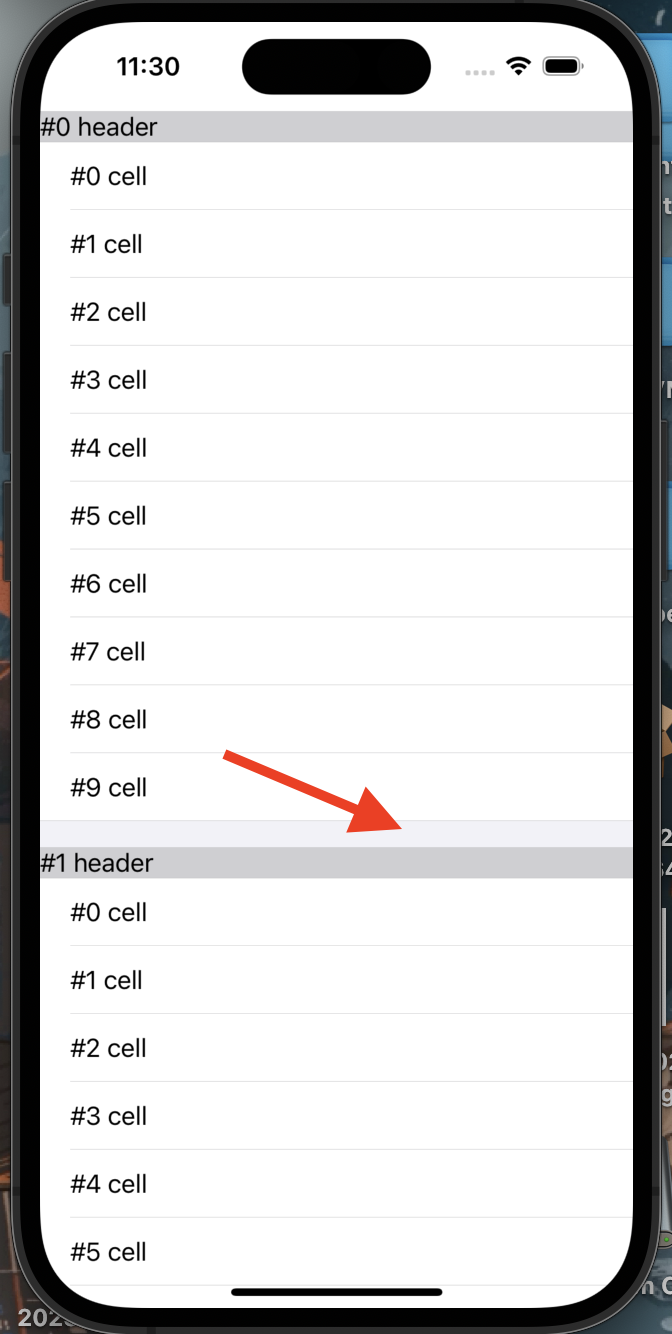
(코드)
import UIKit
class ViewController: UIViewController {
private let tableView: UITableView = {
let view = UITableView(frame: .zero, style: .grouped)
view.allowsSelection = false
view.backgroundColor = .clear
view.separatorStyle = .none
view.bounces = true
view.showsVerticalScrollIndicator = true
view.contentInset = .zero
view.register(UITableViewCell.self, forCellReuseIdentifier: "cell")
view.estimatedRowHeight = 34
view.translatesAutoresizingMaskIntoConstraints = false
return view
}()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(tableView)
NSLayoutConstraint.activate([
tableView.leadingAnchor.constraint(equalTo: view.leadingAnchor),
tableView.trailingAnchor.constraint(equalTo: view.trailingAnchor),
tableView.bottomAnchor.constraint(equalTo: view.bottomAnchor),
tableView.topAnchor.constraint(equalTo: view.safeAreaLayoutGuide.topAnchor),
])
tableView.dataSource = self
tableView.delegate = self
}
}
extension ViewController: UITableViewDataSource, UITableViewDelegate {
// cell
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
10
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath)
cell.textLabel?.text = "#\(indexPath.row) cell"
return cell
}
// header
func numberOfSections(in tableView: UITableView) -> Int {
3
}
func tableView(_ tableView: UITableView, viewForHeaderInSection section: Int) -> UIView? {
let label = UILabel()
label.text = "#\(section) header"
label.backgroundColor = .gray.withAlphaComponent(0.3)
return label
}
}
- footer 영역인걸 확인하기 위해 footer view를 따로 만들고 backgroundColor를 green으로 설정
func tableView(_ tableView: UITableView, viewForFooterInSection section: Int) -> UIView? {
let view = UIView()
view.backgroundColor = .green
return view
}
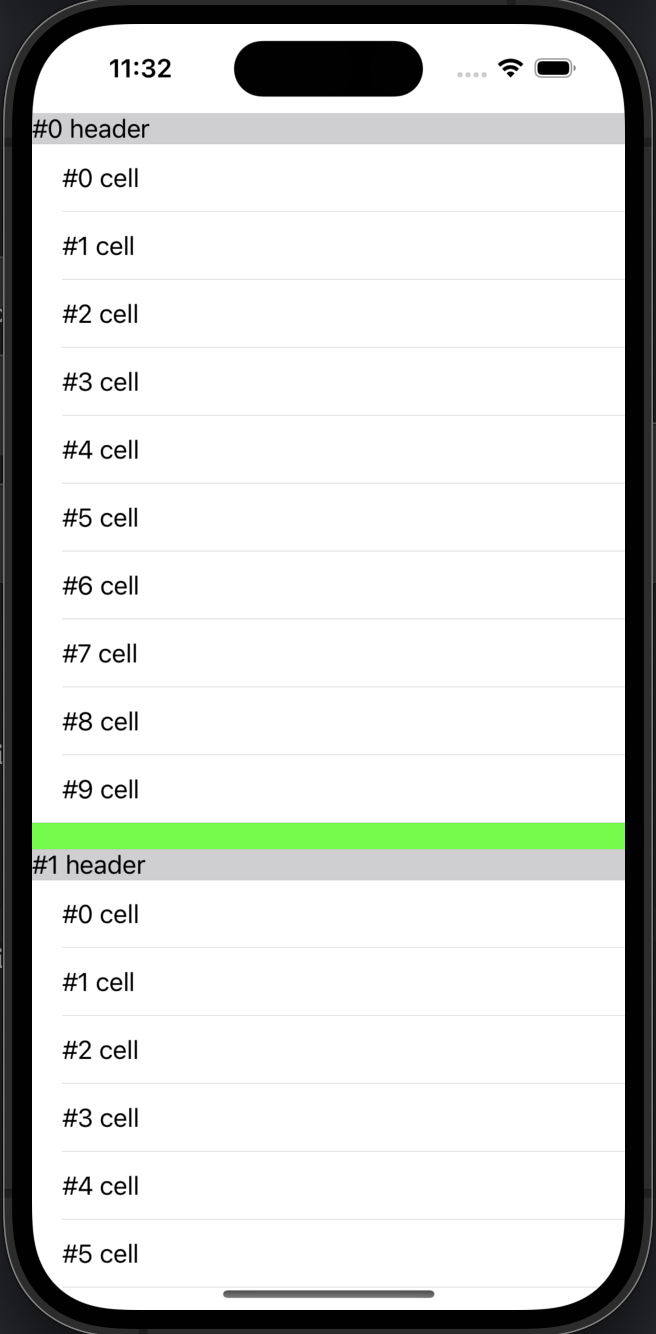
- viewForFooterInSection를 지우고, 아래 코드를 따로 추가하여 footer 영역을 삭제
// footer 영역 삭제
tableView.tableFooterView = UIView(frame: .zero)
tableView.sectionFooterHeight = 0

* 전체 코드: https://github.com/JK0369/ExGrouped
'iOS 응용 (swift)' 카테고리의 다른 글
Comments