Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- swiftUI
- UITextView
- scrollview
- Clean Code
- MVVM
- collectionview
- 스위프트
- HIG
- Human interface guide
- Protocol
- 리펙토링
- tableView
- clean architecture
- ribs
- uitableview
- swift documentation
- 애니메이션
- 리팩토링
- Refactoring
- Xcode
- rxswift
- uiscrollview
- combine
- ios
- UICollectionView
- SWIFT
- 클린 코드
- RxCocoa
- map
- Observable
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] @Sendable 개념, 캡쳐하는 변수를 변경하지 못하게 강제화 하는방법 (동시성, 불변성, concurrency, 동시성 프로그래밍) 본문
iOS 응용 (swift)
[iOS - swift] @Sendable 개념, 캡쳐하는 변수를 변경하지 못하게 강제화 하는방법 (동시성, 불변성, concurrency, 동시성 프로그래밍)
jake-kim 2024. 5. 6. 01:13@Sendable 개념
- Sendable이라는 의미는 "전달 할 수 있는"이라는 의미이지만, 생략된 의미가 존재
- @Sendable는 불변성을 보장하는 "전달 할 수 있는"의 의미로 사용
- 동시성 프로그래밍에서 핵심은 프로퍼티들의 '불변성'을 유지하는 것
- 프로퍼티들이 '불변성'을 만족한다면, 동시성 프로그래밍에서 쉽게 파라미터, 클로저 등에 넘겨서 처리하는 것에 race condition, dead lock, memory conflict 등의 문제등을 신경쓰지 않고 편하게 프로그래밍이 가능
- @Sendable의 목적은 값을 변경할 수 없도록 강제화하는것
예제
- 특정 closure가 있을 때 이 closure에서는 전역변수인 age값 수정이 가능
class ViewController: UIViewController {
var age = 0
var closure: ((_ value: Int) -> Void)?
override func viewDidLoad() {
super.viewDidLoad()
closure = { [weak self] value in
self?.age = 1
}
}
}
- 하지만 @Sendable 키워드를 클로저 안에 사용하면 해당 클로저 안에서는 외부의 값을 수정하지 못함
- 컴파일 에러 발생
class ViewController: UIViewController {
var age = 0
var closure: ((_ value: Int) -> Void)?
override func viewDidLoad() {
super.viewDidLoad()
closure = { @Sendable [weak self] value in
self?.age = 1 // compile error: Main actor-isolated property 'age' can not be mutated from a Sendable closure
}
}
}
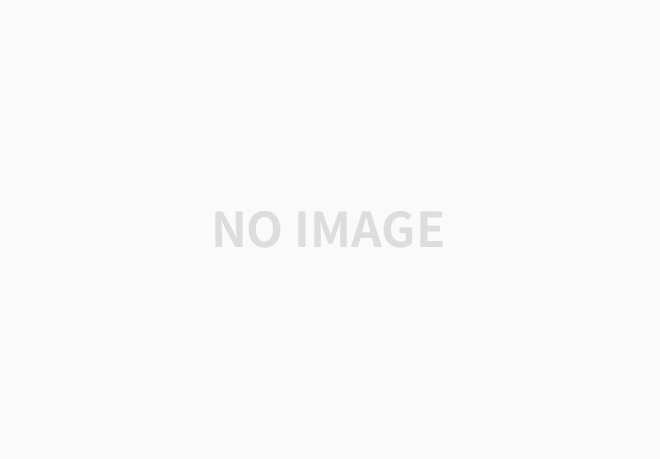
* 참고
'iOS 응용 (swift)' 카테고리의 다른 글
[iOS - swift] consume, consuming 개념 (메모리 효율성, CoW) (0) | 2024.05.10 |
---|---|
[iOS - swift] 2. 클로저를 사용할 때 주의할 점 - nested closure 두 번째 (1) | 2024.05.08 |
[iOS - swift] Dictionary에서 default값 설정 방법 (딕셔너리 디폴트 값) (0) | 2024.05.01 |
[iOS - swift] reduce 연산자 사용 주의사항 (reduce(into:_:), reduce(_:_:), 문자열 사이 콤마 넣기) (0) | 2024.04.29 |
[iOS - swift] 코드를 작성할 때 protocol로 DI 구조를 잘 유지하는 방법 (#성능 이슈) (3) | 2024.04.19 |
Comments
jake-kim님의
글이 좋았다면 응원을 보내주세요!