Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- uitableview
- collectionview
- Human interface guide
- 리팩토링
- HIG
- 스위프트
- Protocol
- MVVM
- ios
- Xcode
- scrollview
- 클린 코드
- swiftUI
- uiscrollview
- RxCocoa
- combine
- Observable
- UITextView
- Clean Code
- tableView
- clean architecture
- ribs
- map
- SWIFT
- UICollectionView
- Refactoring
- 리펙토링
- 애니메이션
- swift documentation
- rxswift
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] 2. collectionView 구현, custom cell 본문
2. collectionView 구현, custom cell
3. collectionView 레이아웃 개념 (UICollectionViewFlowLayout)
programmtically CollectionView 구현
xib사용하여 CollectionView 구현
- CollectionView 추가 -> 구분을 위해 CollectionView와 Cell 배경 색상 세팅
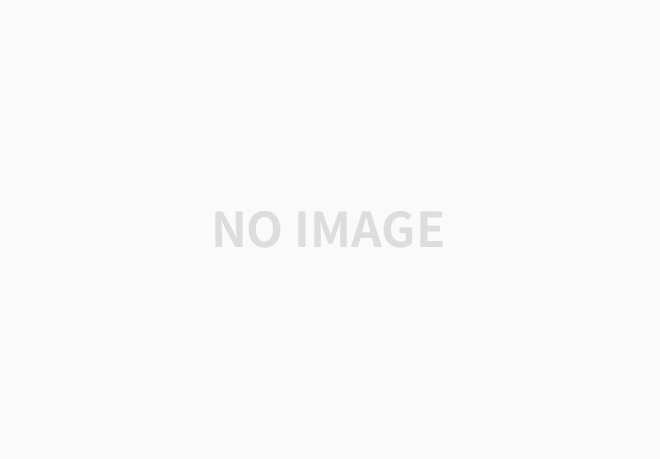
- Cell의 Identifier 설정
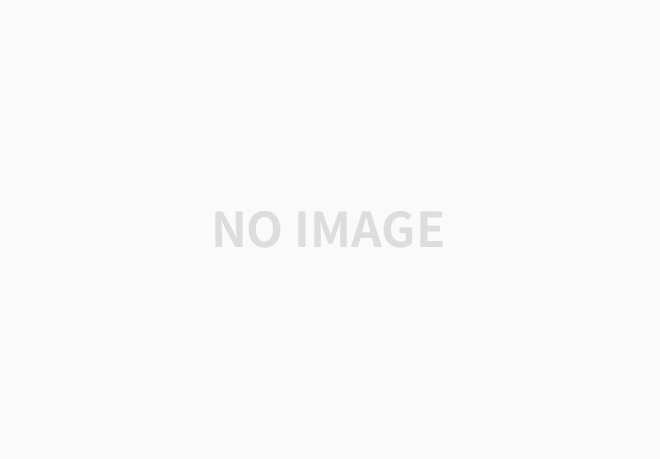
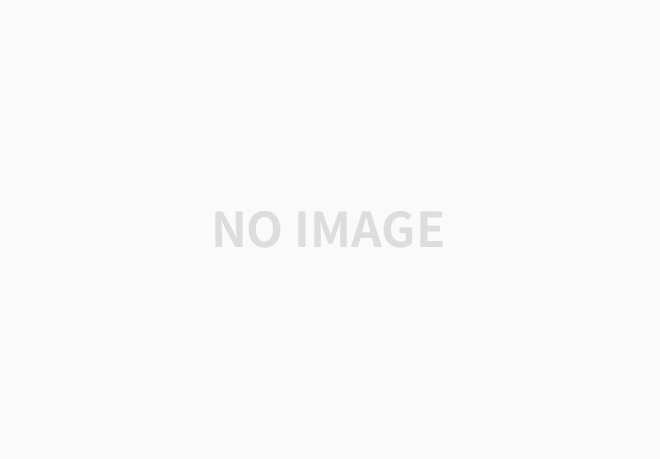
- CollectionView의 DataSource 정의
- collectionView @IBOutlet 설정
- tableView와 동일하게 DataSource에서 numberOfItemsInSection, cellForItemAt 세팅
class ViewController: UIViewController {
@IBOutlet weak var collectionView: UICollectionView!
var numberOfCell = 10
var cellIdentifier = "MyCollectionViewCell"
override func viewDidLoad() {
super.viewDidLoad()
setupDelegate()
}
private func setupDelegate() {
collectionView.dataSource = self
}
}
extension ViewController: UICollectionViewDataSource {
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return numberOfCell
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: cellIdentifier, for: indexPath)
return cell
}
}
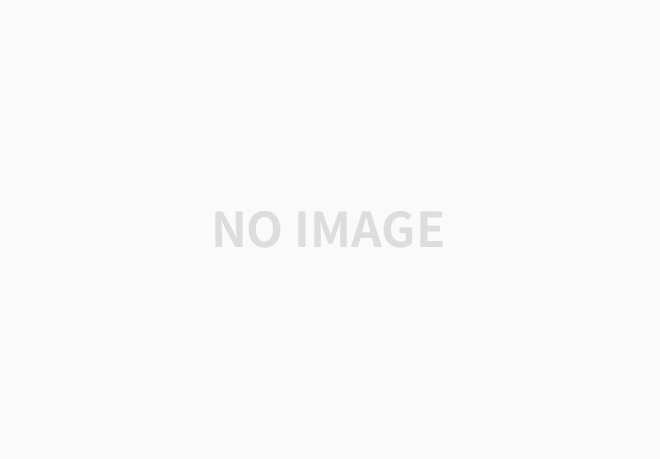
DataSource 업데이트
- TableView와 동일하게 reloadData()호출
- ex) didSelectItemAt에서 아이템 갯수를 늘리고, reloadData() 하도록 설정
collectionView.delegate = self
...
extension ViewController: UICollectionViewDelegate {
func collectionView(_ collectionView: UICollectionView, didSelectItemAt indexPath: IndexPath) {
numberOfCell += 1
collectionView.reloadData()
}
}
Custom Cell 사용 방법
- cell이 기본적으로 가지고 있는 프로퍼티 (code base 작업 시 참고)
var contentView: UIView
var backgroundView: UIView?
var selectedBackgroundView: UIView?
var isSelected: Bool
var isHighlighted: Bool
- 커스텀 셀 UI 작성 - label이 존재하는 커스텀 셀
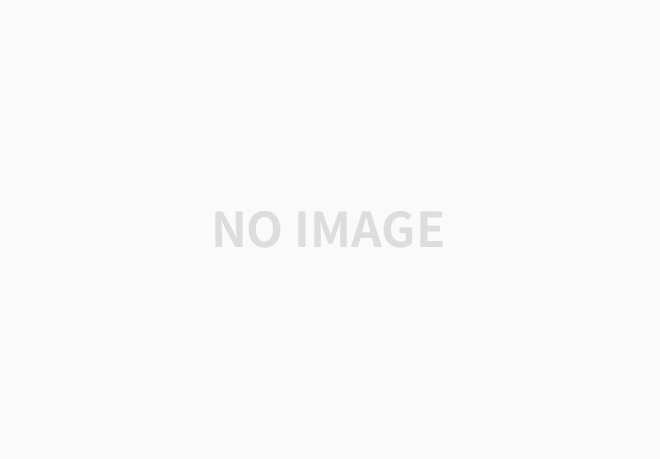
- 커스텀 셀 클래스 생성: 커스텀 셀 자체가 새로운 인스턴스가 되므로 새로운 클래스가 필요
- 만약 위처럼 customCell이 CollectionView안에 내장되어 있지 않으면 코드에서 collectionView.register()를 통해 cell등록이 따로 필요
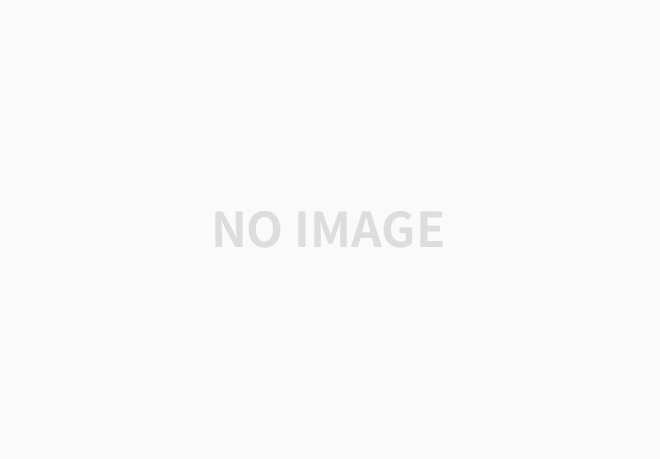
class MyCollectionViewCell: UICollectionViewCell {
@IBOutlet weak var titleLabel: UILabel!
}
- sample dataSouce 세팅
// ViewController.swift
override func viewDidLoad() {
super.viewDidLoad()
setupDelegate()
loadData()
}
private func loadData() {
let sampleData = ["One", "Two", "Three", "four", "Five", "Six", "Seven"]
dataSource = sampleData
}
- cellForItemAt에서 셀에 데이터 입력 및 커스텀 셀 객체 반환
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: MyCollectionViewCell.identifier, for: indexPath) as! MyCollectionViewCell
cell.data = dataSource[indexPath.item]
return cell
}
- 결과
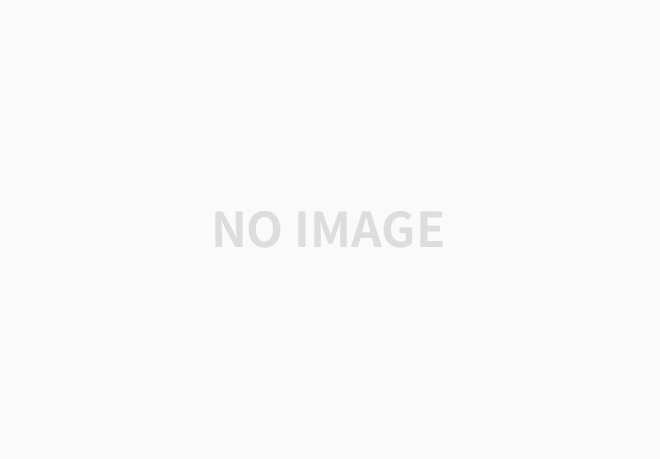
* source code: https://github.com/JK0369/collectionView_sample
* 참고
'iOS 기본 (swift)' 카테고리의 다른 글
[iOS - swift] leading과 left, trailing과 right (0) | 2021.06.05 |
---|---|
[iOS - swift] Archive(아카이브), 아카이빙, 언아카이빙, NSCoding (0) | 2021.06.05 |
[iOS - swift] 1. collectionView 개념 (0) | 2021.06.03 |
[iOS - swift] NotificationCenter (background에서 foreground 진입 이벤트) (0) | 2021.06.02 |
[iOS - swift] SPM(Swift Pacakage Manager) 사용 방법 (0) | 2021.05.22 |