Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- map
- Human interface guide
- 리팩토링
- swiftUI
- UITextView
- uiscrollview
- 리펙토링
- rxswift
- uitableview
- MVVM
- tableView
- Observable
- Clean Code
- collectionview
- 클린 코드
- Refactoring
- ribs
- Xcode
- combine
- swift documentation
- ios
- RxCocoa
- HIG
- Protocol
- scrollview
- 애니메이션
- UICollectionView
- clean architecture
- 스위프트
- SWIFT
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift 공식 문서] 8. Enumerations (열거형) 본문
Enumerations
- enum은 value type
- Enum 네이밍: 복수가 아닌 단수형태이고 대문자로 시작
enum CompassPoint {
case north
case south
case east
case west
}
var directionToHead = CompassPoint.west
CaseIterable
- case들의 사례를 모두 가져오고 싶은 경우 CaseIterable을 conform하여 구현
- 'allCases' 프로퍼티 사용
enum Beverage: CaseIterable {
case coffee, tea, juice
}
let numberOfChoices = Beverage.allCases.count
print("\(numberOfChoices) beverages available")
// Prints "3 beverages available"
print(Beverage.allCases)
// [ProjectName.Beverage.coffee, ProjectName.Beverage.tea, ProjectName.Beverage.juice]
- for문에서 사용
for beverage in Beverage.allCases {
print(beverage)
}
// coffee
// tea
// juice
Associated Value
- case값과 함께 다른 유형의 값을 저장할 수 있다는 점이 Enum에서 유용한 점
- ex) 바코드 표현: 4개의 Int값 or 1개의 문자열이 필요
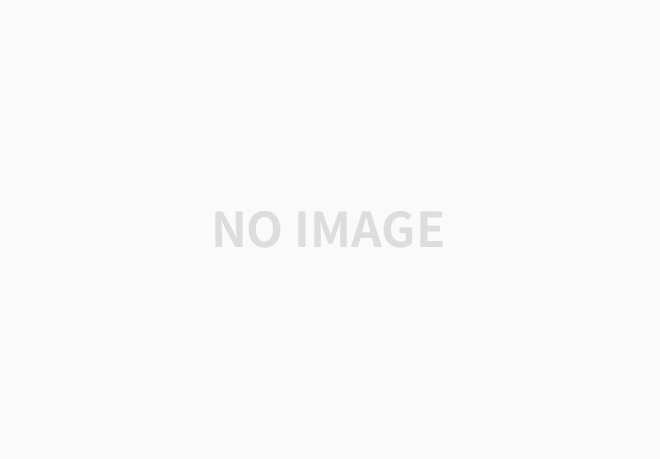
- 바코드를 정의하는 열거형
enum Barcode {
case upc(Int, Int, Int, Int)
case qrCode(String)
}
var productBarcode1 = Barcode.upc(8, 85909, 51226, 3)
var productBarcode2 = .qrCode("ABCDEFGHIJKLMNOP")
- switch 문과 함께 사용
switch productBarcode {
case .upc(let numberSystem, let manufacturer, let product, let check):
print("UPC: \(numberSystem), \(manufacturer), \(product), \(check).")
case .qrCode(let productCode):
print("QR code: \(productCode).")
}
// Prints "QR code: ABCDEFGHIJKLMNOP."
Raw Values
- 열거 case가 서로 다른 유형의 연관된 값을 저장한다고 선언하는 방법
enum ASCIIControlCharacter: Character {
case tab = "\t"
case lineFeed = "\n"
case carriageReturn = "\r"
}
- 암시적 Raw Values: 첫 case에만 raw value할당 시 나머지에도 자동 적용
- Int 형의 Raw Value
- rawValue를 설정하지 않을 경우 첫번째 값은 0
enum Planet: Int {
case mercury = 1, venus, earth, mars, jupiter, saturn, uranus, neptune
}
// venus = 2
// earth = 3
- String형의 Raw Value
- rawValue를 설정하지 않을 경우, case에 선언한 문자열 그대로 입력
enum CompassPoint: String {
case north, south, east, west
}
CompassPoint.north.rawValue // "north"
- rawValue 값으로 초기화
enum CompassPoint: String {
case north, south, east, west
}
let north = CompassPoint(rawValue: "north")
Enum 재귀 - indirect
- case문 앞에 선언
enum ArithmeticExpression {
case number(Int)
indirect case addition(ArithmeticExpression, ArithmeticExpression)
indirect case multiplication(ArithmeticExpression, ArithmeticExpression)
}
- 모든 case 문제 적용 - enum 왼쪽에 선언
// 재귀 enum 정의
indirect enum ArithmeticExpression {
case number(Int)
case addition(ArithmeticExpression, ArithmeticExpression)
case multiplication(ArithmeticExpression, ArithmeticExpression)
}
- 재귀사용
// 값 초기화
let five = ArithmeticExpression.number(5)
let four = ArithmeticExpression.number(4)
let sum = ArithmeticExpression.addition(five, four)
let product = ArithmeticExpression.multiplication(sum, ArithmeticExpression.number(2))
// 사용
func evaluate(_ expression: ArithmeticExpression) -> Int {
switch expression {
case let .number(value):
return value
case let .addition(left, right):
return evaluate(left) + evaluate(right)
case let .multiplication(left, right):
return evaluate(left) * evaluate(right)
}
}
print(evaluate(sum)) // 9
* 참고
https://docs.swift.org/swift-book/LanguageGuide/Enumerations.html
'swift 공식 문서' 카테고리의 다른 글
[iOS - swift 공식 문서] 10. Properties (프로퍼티) (0) | 2021.06.26 |
---|---|
[iOS - swift 공식 문서] 9. Structures and Classes (0) | 2021.06.25 |
[iOS - swift 공식 문서] 7. Closure (클로저) (0) | 2021.06.24 |
[iOS - swift 공식 문서] 6. Functions (함수) (0) | 2021.06.23 |
[iOS - swift 공식 문서] 5. Control flow (흐름 제어) (0) | 2021.06.21 |
Comments
jake-kim님의
글이 좋았다면 응원을 보내주세요!