Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |
Tags
- UICollectionView
- combine
- Refactoring
- scrollview
- SWIFT
- 리팩토링
- swift documentation
- swiftUI
- tableView
- HIG
- uiscrollview
- 애니메이션
- 리펙토링
- Observable
- 스위프트
- collectionview
- map
- RxCocoa
- Clean Code
- uitableview
- ios
- Xcode
- rxswift
- 클린 코드
- MVVM
- Protocol
- ribs
- Human interface guide
- clean architecture
- UITextView
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] Reusable 프레임워크 사용 방법 본문
Reusable 프레임워크
- Cell 등록 시 편의성 향상
- 문자열을 입력하지 않고, 클래스만 입력하여type-safe하므로 컴파일 타임에 오류 감지 가능
- Reusable 미적용 - tableView에 cell을 등록하고 cell을 리턴 할 경우
// cell 등록 tableView.register(MyTableViewCell.self, forCellReuseIdentifier: "MyTableViewCell") // dequeue cell let cell = tableView.dequeueReusableCell(withIdentifier: "MyTableViewCell", for: indexPath) as! MyTableViewCell
- Reusable 적용 - tableView에 cell을 등록하고 cell을 리턴 할 경우
// cell 등록 tableView.register(cellType: MyTableViewCell.self) // dequeue cell let cell = tableView.dequeueReusableCell(for: indexPath) as MyTableViewCell
- Reusable 미적용 - tableView에 cell을 등록하고 cell을 리턴 할 경우
- 문자열로 등록하지 않고 더 간편하며, dequeue cell에서는 강제 unwrapping을 시도하지 않아서 swiftlint에 걸리지 않는 깔끔한 코드
Reusable 사용 방법
- Cell 정의 시 Reusable을 준수
import UIKit import Reusable final class MyTableViewCell: UITableViewCell, Reusable { }
- MyTableViewCell 전체 코드
final class MyTableViewCell: UITableViewCell, Reusable { // MARK: Constants private enum Metric { static let separatorHeight = 10.0 static let gradientViewHeight = 200.0 } // MARK: UI private let titleLabel = UILabel().then { $0.textColor = .black $0.font = UIFont.systemFont(ofSize: 15) } // MARK: Initializers override init(style: UITableViewCell.CellStyle, reuseIdentifier: String?) { super.init(style: style, reuseIdentifier: reuseIdentifier) addSubviews() makeConstraints() } private func addSubviews() { self.contentView.addSubview(titleLabel) } private func makeConstraints() { self.titleLabel.snp.makeConstraints { $0.centerX.centerY.equalTo(self.contentView) } } func setupLabel(title: String) { titleLabel.text = title } @available(*, unavailable) required init?(coder: NSCoder) { fatalError("init(coder:) has not been implemeneted") } }
- register
tableView.register(cellType: MyTableViewCell.self)
- dequeue
func tableView( _ tableView: UITableView, cellForRowAt indexPath: IndexPath ) -> UITableViewCell { let cell = tableView.dequeueReusableCell(for: indexPath) as MyTableViewCell cell.setupLabel(title: dataSource[indexPath.row]) return cell }
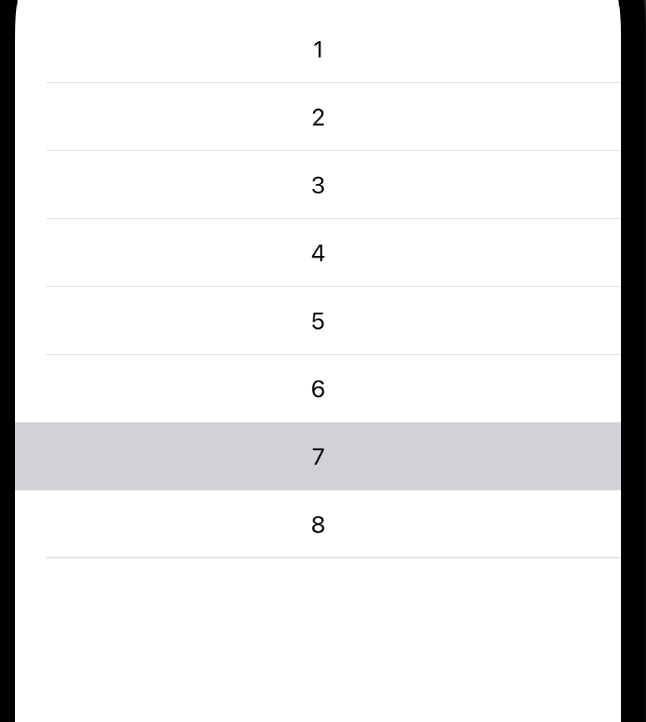
* 전체 소스 코드: https://github.com/JK0369/ExReusable
* 참고
'iOS framework' 카테고리의 다른 글
[iOS - swift] Moya, RxMoya, Networking 레이어 (6) | 2021.12.13 |
---|---|
[iOS - swift] 1. ReactorKit 샘플 앱 - RxDataSources 사용 방법 (0) | 2021.12.10 |
[iOS - swift] 1. Kingfisher 프레임워크 (이미지 캐싱, 이미지 로드) - 사용 방법 (0) | 2021.12.09 |
[iOS - framework] ImagePickerController의 framework (0) | 2020.05.23 |
[iOS - framework] 지도 view controller (길 추적, 이미지 삽입) (0) | 2020.04.30 |