Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |
Tags
- Observable
- swiftUI
- map
- Protocol
- ribs
- 클린 코드
- clean architecture
- collectionview
- uitableview
- Xcode
- UITextView
- Human interface guide
- 리펙토링
- tableView
- 애니메이션
- combine
- Clean Code
- uiscrollview
- Refactoring
- 스위프트
- UICollectionView
- ios
- HIG
- scrollview
- rxswift
- SWIFT
- 리팩토링
- RxCocoa
- swift documentation
- MVVM
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - SwiftUI] GeometryReader, GeometryProxy 사용 방법 (뷰 레이아웃) 본문
iOS 기본 (SwiftUI)
[iOS - SwiftUI] GeometryReader, GeometryProxy 사용 방법 (뷰 레이아웃)
jake-kim 2022. 10. 23. 23:09
*초록색 뷰를 파란색뷰 오른쪽 하단에 위치시키는 방법?
-> 아래에서 알아볼 GeomettryReader를 사용
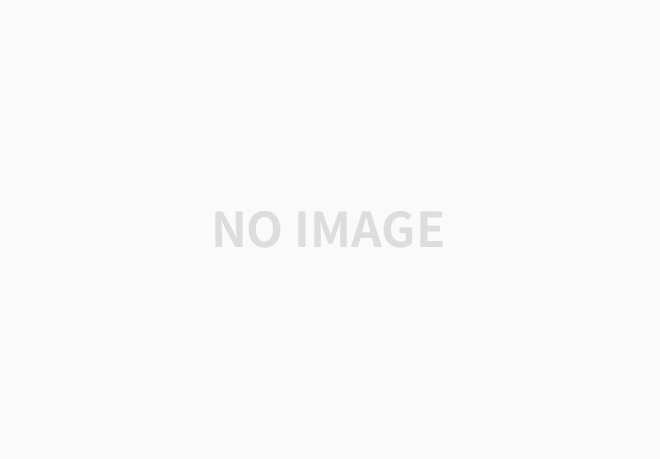
GeometryReader, GeometryProxy 란?
- 뷰의 Container라고 생각하면 되고, 인수로 사용할 수 있는 GeometryProxy가 존재
- GeometryProxy란 좌표와 사이즈 값을 가지고 있는 역할
- 즉, 컨테이너뷰에 관련하여 좌표를 구하고 싶을때나 컨테이너뷰의 크기를 알고 싶은 경우, proxy로 사용
struct ContentView: View {
var body: some View {
VStack {
Text("Jake iOS 앱 개발 알아가기")
GeometryReader { proxy in
}
}
}
}
- GeometryProxy 사용 방법
- size, safeAreaInsets, frame 값을 얻기가 가능
@available(iOS 13.0, macOS 10.15, tvOS 13.0, watchOS 6.0, *)
public struct GeometryProxy {
public var size: CGSize { get }
public subscript<T>(anchor: Anchor<T>) -> T { get }
public var safeAreaInsets: EdgeInsets { get }
public func frame(in coordinateSpace: CoordinateSpace) -> CGRect
}
- frame(in:) 메소드는 CoordinateSpace가 존재
- global: 디바이스의 스크린에 맞춘 좌표
- local: 가장 가까운 부모 뷰와의 상대적인 좌표
- named: 어떤 뷰에든지 .coordinateSpace(name: "someName")이름을 등록할수 있는데, 등록한 뷰에 관한 상대적인 좌표를 구하고 싶을때 사용
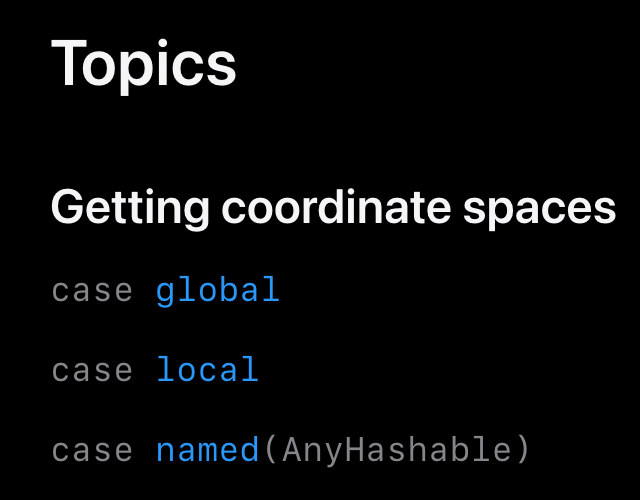
- 코드
var body: some View {
VStack {
Text("Jake iOS 앱 개발 알아가기")
GeometryReader { proxy in
Rectangle()
.foregroundColor(.blue)
.onAppear {
print("size = \(proxy.size)") // (390.0, 730.357)
print("safeAreaInsets = \(proxy.safeAreaInsets)") // EdgeInsets(top: 0.0, leading: 0.0, bottom: 34.0, trailing: 0.0)
// global, local, named
print("global = \(proxy.frame(in: .global))") // (0.0, 79.64241536458333, 390.0, 730.357)
print("local = \(proxy.frame(in: .local))") // (0.0, 0.0, 390.0, 730.357)
print("name = \(proxy.frame(in: .named("OuterVStack")))") // (0.0, 32.64241536458333, 390.0, 730.357)
}
}
.coordinateSpace(name: "OuterVStack")
}
proxy를 이용하여 초록색 뷰 우측하단에 놓기
- 파란 뷰 준비
struct ContentView: View {
var body: some View {
VStack {
Text("Jake iOS 앱 개발 알아가기")
GeometryReader { proxy in
Rectangle()
.foregroundColor(.blue)
}
}
}
}
- .overay를 사용하여 파란 뷰 위에 초록색 뷰를 얹고, proxy의 size, frame값을 이용하여 배치
GeometryReader { proxy in
Rectangle()
.foregroundColor(.blue)
.onAppear {
print("size = \(proxy.size)")
print("safeAreaInsets = \(proxy.safeAreaInsets)")
// global, local, named
print("global = \(proxy.frame(in: .global))")
print("local = \(proxy.frame(in: .local))")
print("name = \(proxy.frame(in: .named("OuterVStack")))")
}
.overlay(
Rectangle()
.foregroundColor(.green)
.frame(width: proxy.size.width/2, height: proxy.size.height/2)
.offset(
x: proxy.frame(in: .local).minX+proxy.size.width/2/2,
y: proxy.frame(in: .local).minY+proxy.size.height/2/2
)
)
}
* 전체 코드: https://github.com/JK0369/ExGeometryReader
* 참고
https://developer.apple.com/documentation/swiftui/coordinatespace
https://developer.apple.com/documentation/swiftui/geometryproxy
https://developer.apple.com/documentation/swiftui/geometryreader
'iOS 기본 (SwiftUI)' 카테고리의 다른 글
[iOS - SwiftUI] UIHostingController 사용 방법 (UIKit에서 SwiftUI 사용 방법) (0) | 2022.10.25 |
---|---|
[iOS - SwiftUI] coordinateSpace(name:) 사용 방법 (0) | 2022.10.24 |
[iOS - SwiftUI] Gradients (Linear, Angular, Radial) 사용 방법 (0) | 2022.10.22 |
[iOS - SwiftUI] ImagePaint 사용 방법 (#패턴 이미지) (0) | 2022.10.21 |
[iOS - SwiftUI] ScaledShape, RotatedShape, OffsetShape, TransformedShape 사용 방법 (0) | 2022.10.20 |