Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |
Tags
- Observable
- clean architecture
- swiftUI
- rxswift
- 리펙토링
- uiscrollview
- UITextView
- SWIFT
- 클린 코드
- RxCocoa
- Protocol
- 리팩토링
- scrollview
- Refactoring
- tableView
- ribs
- HIG
- swift documentation
- uitableview
- 애니메이션
- Xcode
- UICollectionView
- ios
- Clean Code
- map
- combine
- 스위프트
- collectionview
- Human interface guide
- MVVM
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] DispatchQueue 작업 취소, 작업 예약 방법 (DispatchSourceTimer, makeTimerSource, 일시 정지 타이머) 본문
iOS 응용 (swift)
[iOS - swift] DispatchQueue 작업 취소, 작업 예약 방법 (DispatchSourceTimer, makeTimerSource, 일시 정지 타이머)
jake-kim 2024. 10. 7. 01:48DispatchQueue 작업 취소, 예약 방법
- 5초 후에 특정 작업을 수행하고 싶은 경우?
- 보통은 DispatchQueue 사용
DispatchQueue.main.asyncAfter(deadline: .now() + 5) {
// some task...
}
- 5초 후에 특정 작업을 수행하려고 하지만, 중간에 버튼을 누르면 이 작업을 취소하고 싶은 경우?
- DispatchSourceTimer 사용
DispatchSourceTimer 개념
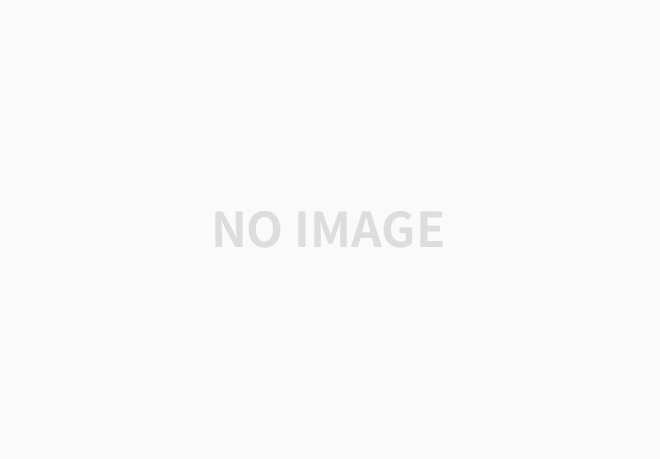
- 타이머를 설정해놓고 특정 작업을 setEventHandler 클로저로 실행시킬 수 있는 기능
- 이것을 사용하면 작업을 등록해놓고 cancel, pause 모두 다 가능
DispatchSourceTimer 사용 방법
- 인스턴스 하나를 두고 cancel(), pause()할 수 있도록 전역으로 선언
class ViewController: UIViewController {
private let dispatchSourceTimer: DispatchSourceTimer = DispatchSource.makeTimerSource(queue: DispatchQueue.main)
}
- viewDidLoad()가 호출되면 작업을 예약
- schedule(deadline:)을 통해 몇초 후에 setEventHandler를 동작시킬지 예약
- setCancelHandler를 통해 취소되었을때 어떤 행동을 할것인지 클로저로 관리
- resume()을 통해 시작
override func viewDidLoad() {
super.viewDidLoad()
runDispatchSourceTimer()
}
func runDispatchSourceTimer() {
dispatchSourceTimer.schedule(deadline: .now() + 5)
dispatchSourceTimer.setEventHandler {
print("event!")
}
dispatchSourceTimer.setCancelHandler {
print("cancel!")
}
dispatchSourceTimer.resume()
}
- 주의사항)
- 5초 후에 setEventHandler가 실행된 후에도 cancel()을 호출하면 setCancelHandler가 실행
- cancel() 메서드를 호출하면 작업이 취소
@objc
private func tap() {
dispatchSourceTimer.cancel()
}
- 기타) schedule(deadline:)은 1회 수행이지만 schedule(deadline:repeating:leeway:)와 같은것을 사용하면 반복적으로 실행가능
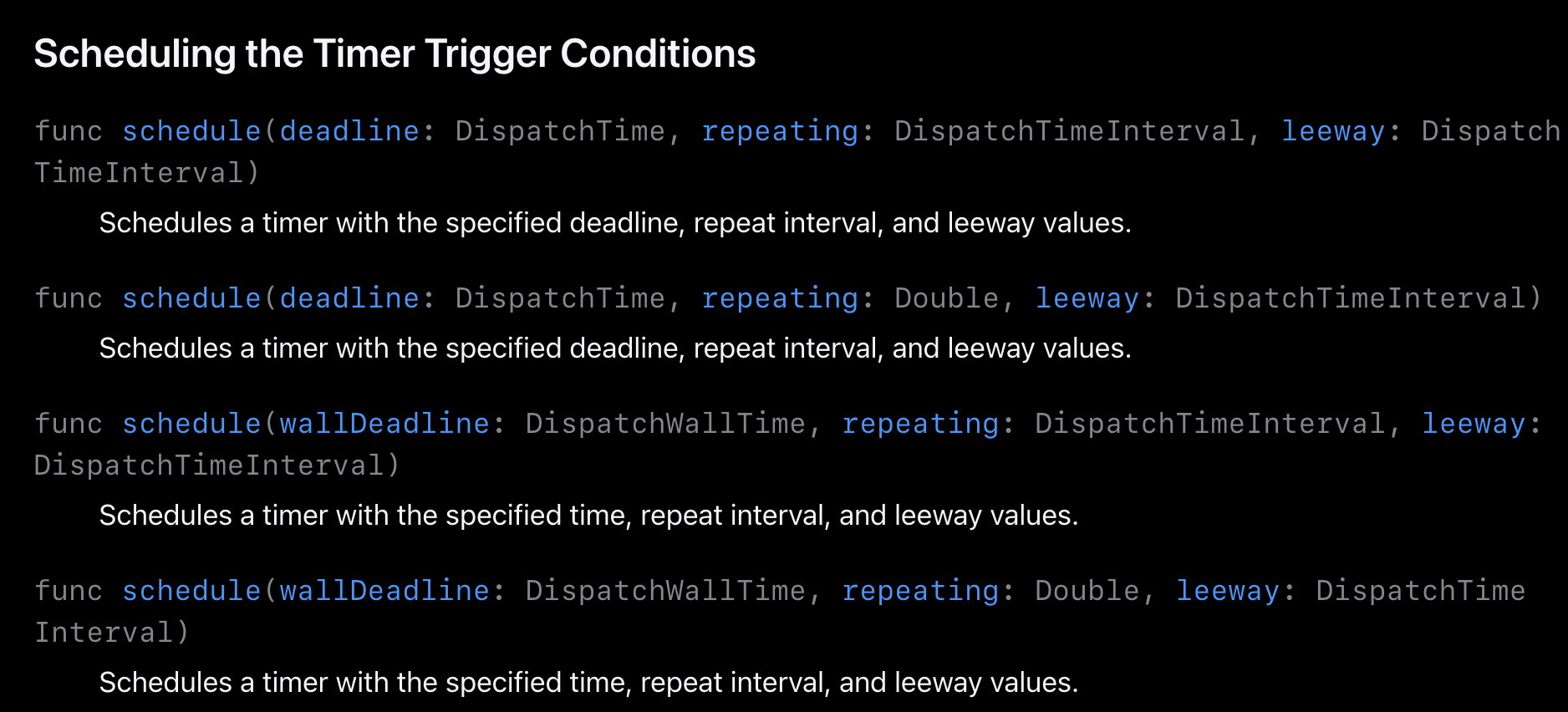
* 전체 코드)
import UIKit
class ViewController: UIViewController {
private let button = {
let btn = UIButton(type: .system)
btn.setTitle("button", for: .normal)
btn.setTitleColor(.blue, for: .normal)
btn.setTitleColor(.systemBlue, for: [.normal, .highlighted])
btn.translatesAutoresizingMaskIntoConstraints = false
return btn
}()
private let dispatchSourceTimer: DispatchSourceTimer = DispatchSource.makeTimerSource(queue: DispatchQueue.main)
override func viewDidLoad() {
super.viewDidLoad()
addButtonLayoutAndEvent()
runDispatchSourceTimer()
}
func addButtonLayoutAndEvent() {
view.addSubview(button)
NSLayoutConstraint.activate([
button.centerXAnchor.constraint(equalTo: view.centerXAnchor),
button.centerYAnchor.constraint(equalTo: view.centerYAnchor),
])
button.addTarget(self, action: #selector(tap), for: .touchUpInside)
}
func runDispatchSourceTimer() {
dispatchSourceTimer.schedule(deadline: .now() + 5)
dispatchSourceTimer.setEventHandler {
print("event!")
}
dispatchSourceTimer.setCancelHandler {
print("cancel!")
}
dispatchSourceTimer.resume()
}
@objc
private func tap() {
dispatchSourceTimer.cancel()
}
}
* 참고
- https://developer.apple.com/documentation/dispatch/dispatchsourcetimer
'iOS 응용 (swift)' 카테고리의 다른 글
[iOS - swift] 디스플레이 확대/축소 옵션 대응 방법 (UIScreen.main.nativeScale) (0) | 2024.10.18 |
---|---|
[iOS - swift] CoreHaptics (진동 주는 방법, 진동 효과) (6) | 2024.10.09 |
[iOS - swift] UIScreenEdgePanGestureRecognizer 개념 (0) | 2024.09.30 |
[iOS - swift] OptionSet으로 flag 리펙토링하기 (플래그 관리, Bool대신 bit 사용하기) (0) | 2024.09.27 |
[iOS - swift] OptionSet에서 시프트 연산자를 사용하는 이유(Shift Operator) (0) | 2024.09.25 |
Comments