Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 리팩토링
- Refactoring
- scrollview
- 스위프트
- ribs
- 클린 코드
- Protocol
- uiscrollview
- 리펙토링
- SWIFT
- swift documentation
- ios
- swiftUI
- collectionview
- RxCocoa
- UITextView
- map
- combine
- Xcode
- 애니메이션
- Clean Code
- Human interface guide
- Observable
- HIG
- uitableview
- rxswift
- tableView
- UICollectionView
- MVVM
- clean architecture
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] String에 substring, removeAt, insertAt 구현 본문
기능
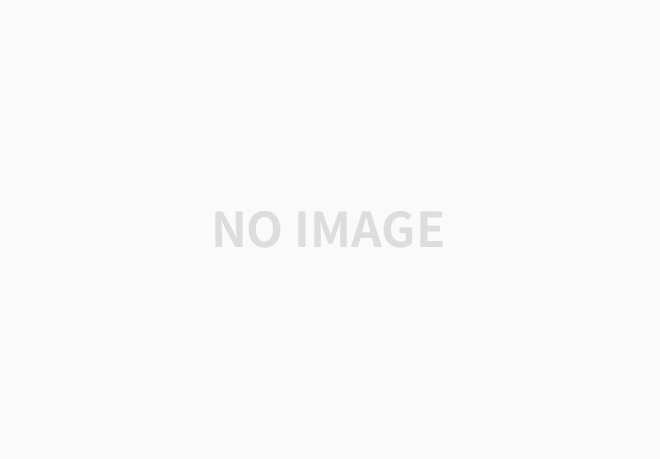
구현 내용
//
// ViewController.swift
// StringProcessing
//
// Created by 김종권 on 2020/12/10.
//
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
print("12345".substring(from: 1, to: 3)) // 234
print("12345".remove(startInd: 2, length: 2)) // 125
print("12345".insertAt(2, string: "7")) // 127345
}
}
extension String {
// from부터 to까지 String
func substring(from: Int?, to: Int?) -> String {
if let start = from {
guard start < self.count else {
return ""
}
}
if let end = to {
guard end >= 0 else {
return ""
}
}
if let start = from, let end = to {
guard end - start >= 0 else {
return ""
}
}
let startIndex: String.Index
if let start = from, start >= 0 {
startIndex = self.index(self.startIndex, offsetBy: start)
} else {
startIndex = self.startIndex
}
let endIndex: String.Index
if let end = to, end >= 0, end < self.count {
endIndex = self.index(self.startIndex, offsetBy: end + 1)
} else {
endIndex = self.endIndex
}
return String(self[startIndex ..< endIndex])
}
// startInd부터 length만큼의 문자열 삭제
func remove(startInd: Int, length: Int) -> String {
return self.substring(from: 0, to: startInd - 1) + self.substring(from: startInd + length, to: self.count - 1)
}
// positino인덱스에 string문자열 삽입
func insertAt(_ position: Int, string: String) -> String {
self.substring(from: 0, to: position - 1) + string + self.substring(from: position, to: self.count - 1)
}
}
'iOS 응용 (swift)' 카테고리의 다른 글
[iOS - swift] 서버 푸시 (remote notification), APNs (Apple Push Notification service) (0) | 2020.12.17 |
---|---|
[iOS - swift] 앱을 첫 번째 실행 시, keychain정보를 삭제하는 방법 (0) | 2020.12.17 |
[iOS - swift] UITextField 포맷 (핸드폰 번호, 이메일, 카드 번호) - AnyFormatKit 사용 (0) | 2020.12.09 |
[iOS - swift] timer 구현 (background에서 다시 foreground로 온 경우에도 적용) (0) | 2020.11.30 |
[iOS - swift] 커스텀 팝업 창 (custom popup, custom alert view) (5) | 2020.11.28 |
Comments