Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- Human interface guide
- 리팩토링
- UITextView
- UICollectionView
- uiscrollview
- swift documentation
- tableView
- 스위프트
- combine
- Observable
- Refactoring
- map
- collectionview
- SWIFT
- 리펙토링
- 클린 코드
- swiftUI
- rxswift
- uitableview
- HIG
- scrollview
- ribs
- Xcode
- RxCocoa
- clean architecture
- ios
- 애니메이션
- Protocol
- MVVM
- Clean Code
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] 선 그리기 (UIBezierPath, CAShapeLayer) 본문
UIBezierPath란
- Bezier(베지에 곡선 할 때 베지에): 선분을 그릴 때 사용
CAShapeLayer란
- CoreAnimation이라 하여 CAShapeLayer 객체를 이용하여 UIBezierPath의 색깔들을 바꿀 수 있고 view.layer에 추가될 수 있는 객체
원리
- UIBezierPath객체로 선분을 그려줌
- CAShapeLayer의 path에 위에서 만든 UIBezierPath객체.cgPath를 등록
- CAShapeLayer로 스타일을 지정해준 뒤, view.layer.addSublayer(CASharpeLayer객체)로 추가
예제) UITextField 밑에 선분 그리기
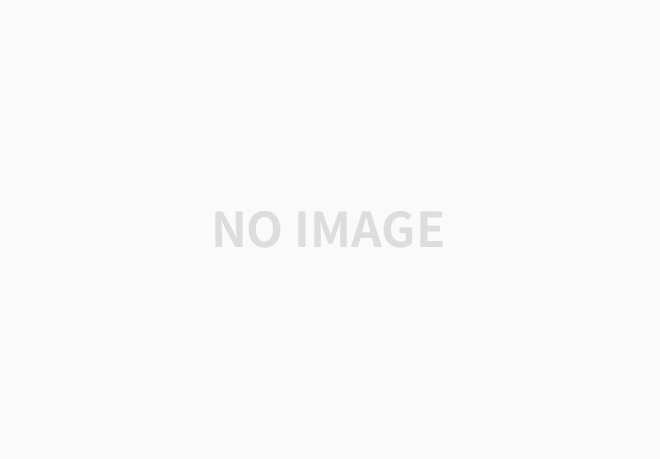
- UIBezierPath의 move(to:) 함수: 그려지는 시작 점 정의
- UIBezierPath의 addLine(to:) 함수: 끝 점 정의
- UIBezierPath의 close() 함수: 그리는 것을 끝내는 의미
class BottomLineTextField: UITextField {
func addBottomView() {
let path = UIBezierPath()
path.move(to: CGPoint(x: 0, y: bounds.size.height + 3))
path.addLine(to: CGPoint(x: bounds.width, y: bounds.size.height + 3))
path.close()
}
}
- CAShapeLayer는 외부에서 접근 할 수 있도록 (텍스트필드 입력에 따라, 밑 라인의 색깔 변화같은 것을 주기 위하여) 전역에 선언
class BottomLineTextField: UITextField {
public var shapeLayer: CAShapeLayer! // 전역에 선언
func addBottomView() {
let path = UIBezierPath()
path.move(to: CGPoint(x: 0, y: bounds.size.height + 3))
path.addLine(to: CGPoint(x: bounds.width, y: bounds.size.height + 3))
path.close()
let shapeLayer = CAShapeLayer()
}
}
- CAShapeLayer에 path.cgPath추가 -> 스타일 정의 -> layer.addSublayer
func addBottomView() {
borderStyle = .none
let path = UIBezierPath()
path.move(to: CGPoint(x: 0, y: bounds.size.height + 3))
path.addLine(to: CGPoint(x: bounds.width, y: bounds.size.height + 3))
path.close()
let shapeLayer = CAShapeLayer()
shapeLayer.path = path.cgPath
shapeLayer.lineWidth = path.lineWidth
shapeLayer.fillColor = UIColor.blue.cgColor
shapeLayer.strokeColor = UIColor.blue.cgColor
layer.addSublayer(shapeLayer)
}
* 전체 코드
//
// ViewController.swift
// path
//
// Created by 김종권 on 2020/12/17.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var textField: BottomLineTextField!
override func viewDidLoad() {
super.viewDidLoad()
textField.addBottomView()
}
}
class BottomLineTextField: UITextField {
public var shapeLayer: CAShapeLayer! // 전역에 선언
func addBottomView() {
borderStyle = .none
let path = UIBezierPath()
path.move(to: CGPoint(x: 0, y: bounds.size.height + 3))
path.addLine(to: CGPoint(x: bounds.width, y: bounds.size.height + 3))
path.close()
let shapeLayer = CAShapeLayer()
shapeLayer.path = path.cgPath
shapeLayer.lineWidth = path.lineWidth
shapeLayer.fillColor = UIColor.blue.cgColor
shapeLayer.strokeColor = UIColor.blue.cgColor
layer.addSublayer(shapeLayer)
}
}
'iOS 실전 (swift)' 카테고리의 다른 글
Comments