Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- scrollview
- rxswift
- 스위프트
- swift documentation
- 리펙토링
- MVVM
- 클린 코드
- SWIFT
- swiftUI
- map
- ribs
- Protocol
- uitableview
- tableView
- Human interface guide
- UICollectionView
- UITextView
- combine
- 애니메이션
- HIG
- RxCocoa
- Observable
- collectionview
- 리팩토링
- ios
- Xcode
- uiscrollview
- Refactoring
- clean architecture
- Clean Code
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] UI 컴포넌트 - GradientView (흐려지는 뷰) 본문
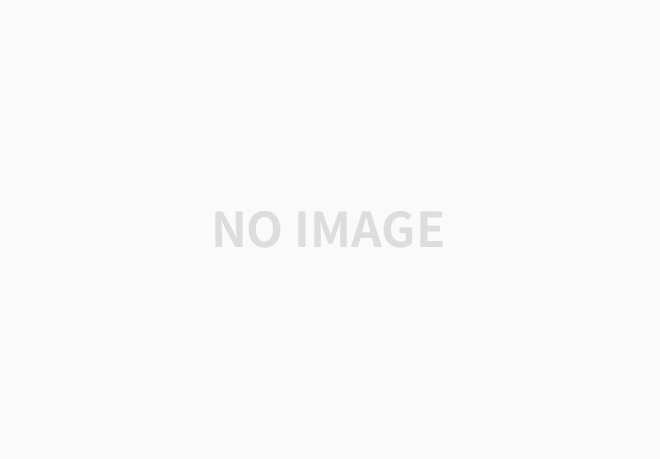
GradientView
- UIView를 상속받아서 구현
- layer.addSublayer로 CAGradientLayer 객체를 추가한 형태
- 특정 layout 또는 특정 component위에 gradientView 객체를 올려서 사용
- isUserInteractionEnabled = false인 상태로 터치 이벤트는 responder chain을 통해 sublayer에 넘기도록 설정
GradientView 구현
- BaseView 정의
class BaseView: UIView {
override init(frame: CGRect) {
super.init(frame: frame)
configure()
}
@available(*, unavailable)
required init?(coder: NSCoder) {
fatalError("required init?(coder: NSCoder) has not been implemented")
}
func configure() {}
func bind() {}
}
- GradientView 클래스 생성
class GradientView: BaseView {
}
- Gradient 방향 정의
enum GradientDirection {
case vertical
case horizontal
}
var direction: GradientDirection = .horizontal
private let gradientLayer = CAGradientLayer()
- gradientLayerColors 정의: gradientLayer에 color를 적용할 경우 2개 이상의 color값이 필요하는데, 이곳에 사용
var gradientLayerColors: [UIColor]? {
didSet { bind() }
}
- convenience init 구현: 초기화 시 direction을 설정할 수 있는 기능 제공
convenience init(direction: GradientDirection) {
self.init(frame: .zero)
self.direction = direction
}
- 해당 뷰 layer에 gradientLayer 객체 추가 및 interaction 비활성화
override func configure() {
super.configure()
layer.addSublayer(gradientLayer)
isUserInteractionEnabled = false
}
- layoutSublayers(of:)에 layer 설정 값 부여
- layoutSublayers(of:)란? autolayout과 같이 layout이 지정될 때와 같이 layer의 bounds가 바뀌면 호출되는 메서드
override func layoutSublayers(of layer: CALayer) {
super.layoutSublayers(of: layer)
switch direction {
case .horizontal:
gradientLayer.startPoint = CGPoint(x: 0.0, y: 0.0)
gradientLayer.endPoint = CGPoint(x: 1.0, y: 0.0)
case .vertical:
gradientLayer.startPoint = CGPoint(x: 0, y: 0)
gradientLayer.endPoint = CGPoint(x: 0, y: 1.0)
}
gradientLayer.frame = bounds
}
- bind(): gradientLayers객체의 color 설정
override func bind() {
super.bind()
gradientLayer.colors = gradientLayerColors?.compactMap { $0.cgColor }
}
- GradientView를 사용하는 쪽: gradientLayerColors를 설정하여 흐려지는 효과 부여
- 해당 gradientView를 흐려지게 하고싶은 view위에 덮거나, 흐려지는 구역에 해당 뷰를 위치시키면 완성
// ViewController.swift
lazy var gradientView: GradientView = {
let view = GradientView()
view.gradientLayerColors = [UIColor.white.withAlphaComponent(0.01), .white]
return view
}()
GradientView 전체 코드
import UIKit
class GradientView: BaseView {
enum GradientDirection {
case vertical
case horizontal
}
var gradientLayerColors: [UIColor]? {
didSet { bind() }
}
var direction: GradientDirection = .horizontal
private let gradientLayer = CAGradientLayer()
convenience init(direction: GradientDirection) {
self.init(frame: .zero)
self.direction = direction
}
override func configure() {
super.configure()
layer.addSublayer(gradientLayer)
isUserInteractionEnabled = false
}
override func layoutSublayers(of layer: CALayer) {
super.layoutSublayers(of: layer)
switch direction {
case .horizontal:
gradientLayer.startPoint = CGPoint(x: 0.0, y: 0.0)
gradientLayer.endPoint = CGPoint(x: 1.0, y: 0.0)
case .vertical:
gradientLayer.startPoint = CGPoint(x: 0, y: 0)
gradientLayer.endPoint = CGPoint(x: 0, y: 1.0)
}
gradientLayer.frame = bounds
}
override func bind() {
super.bind()
gradientLayer.colors = gradientLayerColors?.compactMap { $0.cgColor }
}
}
* 사용 source code: https://github.com/JK0369/GradientViewSample
'UI 컴포넌트 (swift)' 카테고리의 다른 글
Comments
jake-kim님의
글이 좋았다면 응원을 보내주세요!