Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- Refactoring
- Clean Code
- map
- 리팩토링
- collectionview
- 클린 코드
- MVVM
- clean architecture
- 스위프트
- HIG
- uiscrollview
- Observable
- swift documentation
- 애니메이션
- swiftUI
- SWIFT
- ios
- ribs
- Xcode
- scrollview
- UICollectionView
- tableView
- uitableview
- Human interface guide
- rxswift
- combine
- 리펙토링
- Protocol
- UITextView
- RxCocoa
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] 2. CollectionView (컬렉션 뷰) - UICollectionViewFlowLayout을 이용한 CarouselView (수평 스크롤 뷰) 본문
iOS 응용 (swift)
[iOS - swift] 2. CollectionView (컬렉션 뷰) - UICollectionViewFlowLayout을 이용한 CarouselView (수평 스크롤 뷰)
jake-kim 2021. 7. 19. 23:56cf) ScrollView + StackView를 이용한 수평 스크롤 뷰: https://ios-development.tistory.com/617
[iOS - swift] UI 컴포넌트 - 수평 스크롤 뷰 (ScrollView + StackView)
cf) collectionView를 이용한 수평 스크롤 뷰: https://ios-development.tistory.com/632 [iOS - swift] CollectionView(컬렉션 뷰) - 수평 스크롤 뷰 (UICollectionViewFlowLayout) 1. custom layout이 아닌 UICo..
ios-development.tistory.com
1. CollectionView (컬렉션 뷰) - UICollectionViewFlowLayout
2. CollectionView (컬렉션 뷰) - UICollectionViewFlowLayout을 이용한 CarouselView (수평 스크롤 뷰)
3. CollectionView (컬렉션 뷰) - custom layout (grid, pinterest 레이아웃 구현)
4. CollectionView (컬렉션 뷰) -실전 사용 방법 (FlowLayout, CustomLayout, binary search, cache)
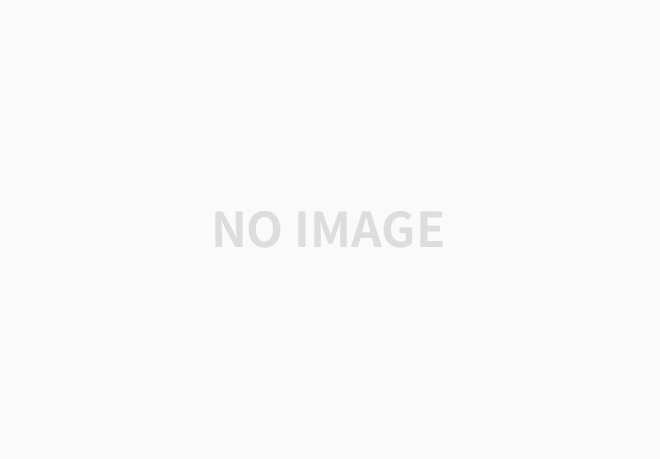
CollectionView에 사용 될 Cell 정의
class MyCell: UICollectionViewCell {
static var id: String { NSStringFromClass(Self.self).components(separatedBy: ".").last ?? "" }
var model: String? { didSet { bind() } }
lazy var titleLabel: UILabel = {
let label = UILabel()
return label
}()
override init(frame: CGRect) {
super.init(frame: frame)
addSubviews()
configure()
}
@available(*, unavailable)
required init?(coder: NSCoder) {
fatalError()
}
private func addSubviews() {
addSubview(titleLabel)
}
private func configure() {
titleLabel.snp.makeConstraints { make in
make.center.equalToSuperview()
}
backgroundColor = .placeholderText
}
private func bind() {
titleLabel.text = model
}
}
CollectionView를 사용할 ViewController에 초기화
- UICollectionViewFlowLayout()을 사용하여 collectionView 초기화
- 핵심은 scrollDirection = .horizontal 설정
class ViewController: UIViewController {
var dataSource: [String] = []
lazy var collectionView: UICollectionView = {
let flowLayout = UICollectionViewFlowLayout()
flowLayout.scrollDirection = .horizontal
flowLayout.minimumLineSpacing = 50 // cell사이의 간격 설정
let view = UICollectionView(frame: .zero, collectionViewLayout: flowLayout)
view.backgroundColor = .brown
return view
}()
}
- viewDidLoad에서 호출 될 샘플 dataSource 세팅
private func setupDataSource() {
for i in 0...10 {
dataSource += ["\(i)"]
}
}
- viewDidLoad에서 호출 될 뷰 추가
private func addSubviews() {
view.addSubview(collectionView)
}
- collectionView의 delegate 할당
private func setupDelegate() {
collectionView.delegate = self
collectionView.dataSource = self
}
- collectionView에 cell 등록
private func registerCell() {
collectionView.register(MyCell.self, forCellWithReuseIdentifier: MyCell.id)
}
- collectionView layout 설정
private func configure() {
collectionView.snp.makeConstraints { make in
make.center.leading.trailing.equalToSuperview()
make.height.equalTo(320)
}
}
collectionView Delegate 구현
- dataSource 카운트, cell 초기화
extension ViewController: UICollectionViewDelegate, UICollectionViewDataSource {
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return dataSource.count
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: MyCell.id, for: indexPath)
if let cell = cell as? MyCell {
cell.model = dataSource[indexPath.item]
}
return cell
}
}
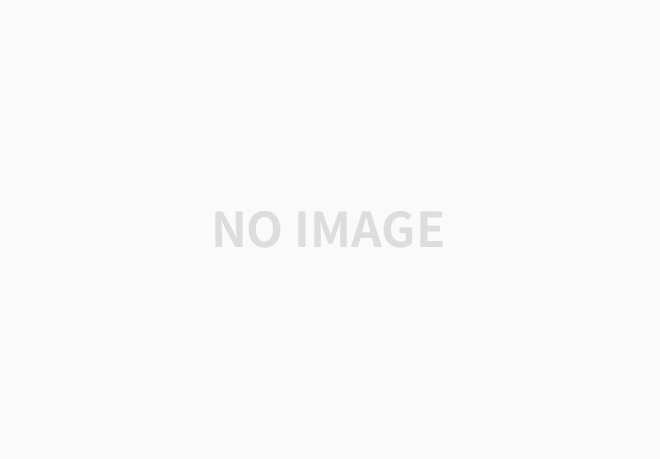
- cell의 height값을 크게하여, cell이 밑이 아닌 오른쪽으로 쭉 뻗어지도록 설정
- UICollectionViewDelegateFlowLayout에서 height값을 collectionView의 height만큼 설정
extension ViewController: UICollectionViewDelegateFlowLayout {
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
return CGSize(width: 320, height: collectionView.frame.height)
}
}
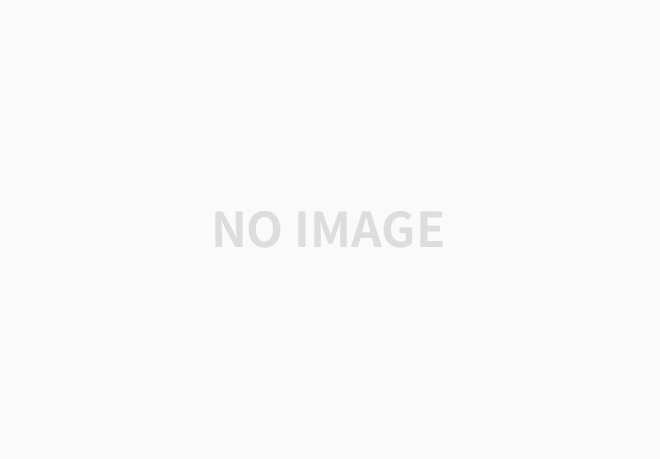
* source code: https://github.com/JK0369/CarouselViewSample
'iOS 응용 (swift)' 카테고리의 다른 글
[iOS - swift] TableView - section, header, footer 사용방법 (0) | 2021.07.27 |
---|---|
[iOS - swift] 3. CollectionView (컬렉션 뷰) - custom layout (grid, pinterest 레이아웃 구현) (4) | 2021.07.20 |
[iOS - swift] 1. CollectionView (컬렉션 뷰) - UICollectionViewFlowLayout (0) | 2021.07.16 |
[iOS - swift] PageViewController (페이지 뷰 컨트롤러) (7) | 2021.07.15 |
[iOS - swift] @available(*, unavailable) 사용하여 비가용성 정의 (0) | 2021.07.13 |