Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- UITextView
- Xcode
- clean architecture
- 스위프트
- MVVM
- ios
- ribs
- uiscrollview
- 리펙토링
- scrollview
- map
- rxswift
- Clean Code
- tableView
- RxCocoa
- SWIFT
- Human interface guide
- UICollectionView
- collectionview
- 애니메이션
- 리팩토링
- combine
- swiftUI
- Protocol
- uitableview
- 클린 코드
- HIG
- Refactoring
- swift documentation
- Observable
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] collectionView의 performBatchUpdates(_:completion:) 본문
iOS 응용 (swift)
[iOS - swift] collectionView의 performBatchUpdates(_:completion:)
jake-kim 2021. 8. 29. 11:15CollectionView의 연산 3가지
- insert, move, delete
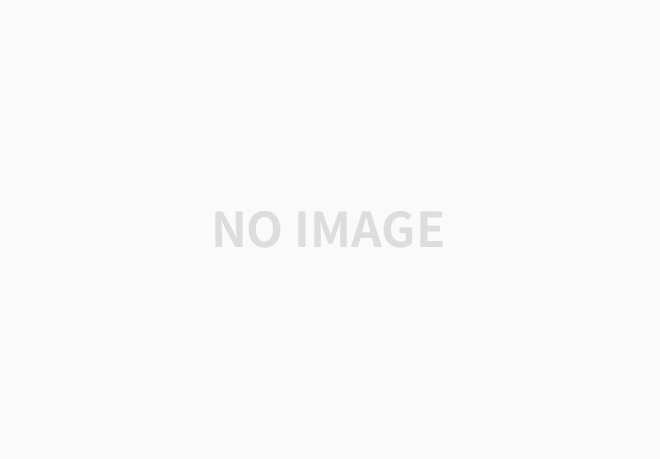
- 3가지 연산의 dicussion에 모두 performBatchUpdates를 사용하여 각 연산의 변경을 동시에 animate를 줄 수 있는 방법
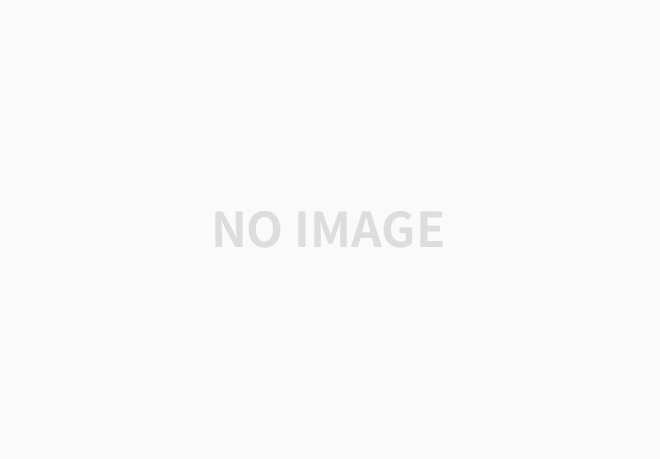
performBatchUpdates(_:completion:)
- 여러개의 변경 후 completion 블럭을 사용할 수 있기 때문에 사용
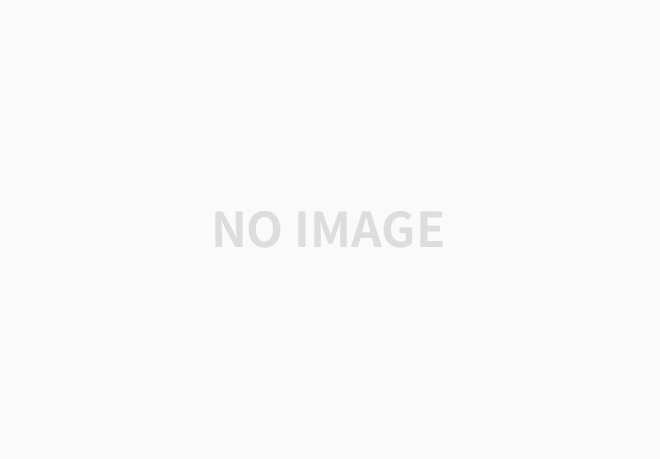
- 코드의 순서에 상관 없이 insert 연산전에 delete 연산이 수행됨을 주의 > 삭제 후 index값 기준으로 index가 처리되므로 주의
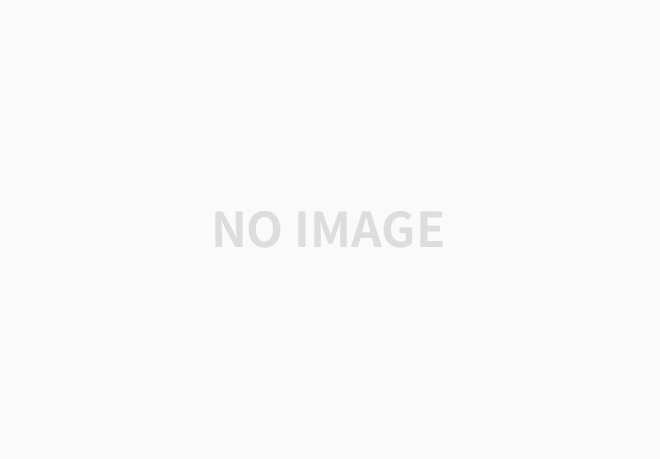
performBatchUpdates(_:completion:) 사용
- collectionView 준비
class ViewController: UIViewController {
// Views
lazy var collectionView: UICollectionView = {
let view = UICollectionView(frame: .zero, collectionViewLayout: UICollectionViewFlowLayout())
view.backgroundColor = .systemGray
view.register(MyCollectionViewCell.self, forCellWithReuseIdentifier: "cell")
view.delegate = self
view.dataSource = self
return view
}()
lazy var stackView: UIStackView = {
let view = UIStackView()
view.spacing = 12.0
view.backgroundColor = .systemGreen
return view
}()
lazy var insertButton: UIButton = {
let button = UIButton()
button.setTitle("삽입", for: .normal)
button.setTitleColor(.blue, for: .normal)
button.addTarget(self, action: #selector(didTapInsertButton(_:)), for: .touchUpInside)
return button
}()
lazy var deleteButton: UIButton = {
let button = UIButton()
button.setTitle("삭제", for: .normal)
button.setTitleColor(.blue, for: .normal)
button.addTarget(self, action: #selector(didTapDeleteButton(_:)), for: .touchUpInside)
return button
}()
lazy var moveButton: UIButton = {
let button = UIButton()
button.setTitle("이동", for: .normal)
button.setTitleColor(.blue, for: .normal)
button.addTarget(self, action: #selector(didTapMoveButton(_:)), for: .touchUpInside)
return button
}()
// Controls
var dataSource = ["1", "2", "3", "4", "5", "6"]
override func viewDidLoad() {
super.viewDidLoad()
setupView()
setupLayout()
}
func setupView() {
view.backgroundColor = .systemBackground
view.addSubview(collectionView)
view.addSubview(stackView)
[insertButton, deleteButton, moveButton].forEach{ stackView.addArrangedSubview($0) }
}
func setupLayout() {
collectionView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activate([
collectionView.topAnchor.constraint(equalTo: view.safeAreaLayoutGuide.topAnchor, constant: 56),
collectionView.leadingAnchor.constraint(equalTo: view.leadingAnchor),
collectionView.trailingAnchor.constraint(equalTo: view.trailingAnchor),
collectionView.bottomAnchor.constraint(equalTo: view.safeAreaLayoutGuide.bottomAnchor, constant: -120)
])
stackView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activate([
stackView.centerXAnchor.constraint(equalTo: view.centerXAnchor),
stackView.bottomAnchor.constraint(equalTo: view.safeAreaLayoutGuide.bottomAnchor, constant: -36)
])
}
@objc func didTapInsertButton(_ sender: Any) {
dataSource.insert("123", at: 0)
collectionView.insertItems(at: [IndexPath(item: 0, section: 0)])
dataSource.remove(at: 6)
collectionView.deleteItems(at: [IndexPath(item: 6, section: 0)])
}
@objc func didTapDeleteButton(_ sender: Any) {
}
@objc func didTapMoveButton(_ sender: Any) {
}
}
extension ViewController: UICollectionViewDelegate, UICollectionViewDataSource {
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return dataSource.count
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cell", for: indexPath) as! MyCollectionViewCell
cell.text = dataSource[indexPath.item]
return cell
}
}
- performBatchUpdates(:completion:)없이 insert, delete 구현
// 현재 데이터 소스는 6개있지만, 한개를 추가하고 (7개), 6번째 인덱스에 있는 값 삭제
@objc func didTapInsertButton(_ sender: Any) {
dataSource.insert("123", at: 0)
collectionView.insertItems(at: [IndexPath(item: 0, section: 0)])
dataSource.remove(at: 6)
collectionView.deleteItems(at: [IndexPath(item: 6, section: 0)])
}
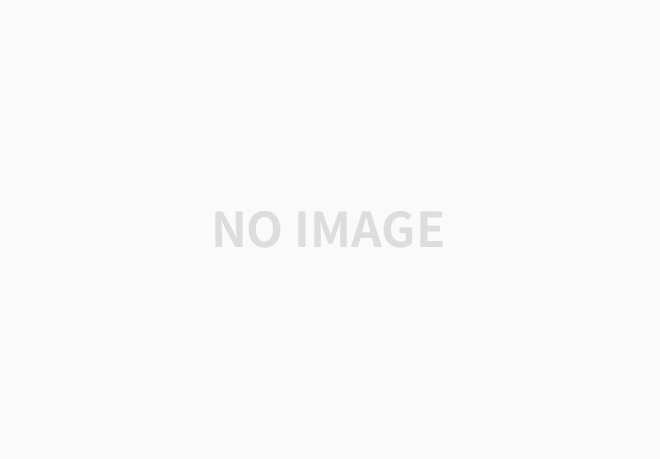
- performBatchUpdates(:completion:) 사용: 실행 화면은 동일
// runtime error: remove가 먼저 되므로, 지금 index는 5가 최대인 상태라 에러 발생
@objc func didTapInsertButton(_ sender: Any) {
collectionView.performBatchUpdates {
dataSource.insert("123", at: 0)
collectionView.insertItems(at: [IndexPath(item: 0, section: 0)])
dataSource.remove(at: 6)
collectionView.deleteItems(at: [IndexPath(item: 6, section: 0)])
} completion: { [weak self] _ in
print(self?.collectionView.numberOfItems(inSection: 0))
}
}
// 정상 코드
@objc func didTapInsertButton(_ sender: Any) {
collectionView.performBatchUpdates {
dataSource.insert("123", at: 0)
collectionView.insertItems(at: [IndexPath(item: 0, section: 0)])
dataSource.remove(at: 5)
collectionView.deleteItems(at: [IndexPath(item: 5, section: 0)])
} completion: { [weak self] _ in
print(self?.collectionView.numberOfItems(inSection: 0))
}
}
- performBatchUpdates(:completion:) 사용 시 이점: completion 활용 가능
* 전체 코드: https://github.com/JK0369/collectionViewEx_
* 참고
- https://developer.apple.com/documentation/uikit/uicollectionview
- https://developer.apple.com/documentation/uikit/uicollectionview/1618045-performbatchupdates