Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |
Tags
- Refactoring
- SWIFT
- UITextView
- ios
- combine
- uiscrollview
- ribs
- UICollectionView
- 스위프트
- MVVM
- 애니메이션
- rxswift
- 리펙토링
- Xcode
- RxCocoa
- 클린 코드
- swift documentation
- 리팩토링
- HIG
- tableView
- swiftUI
- collectionview
- clean architecture
- uitableview
- Clean Code
- Protocol
- map
- Human interface guide
- Observable
- scrollview
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] allSatisfy 연산자 (Collection, Sequence 개념) 본문
allSatisfy 연산자
- Collection의 모든 요소가 특정 조건을 만족시키는지 알고 싶은 경우 사용
- ex) 배열을 순회하면서 원소들이 특정 조건을 모두 만족하는지 확인할 때 사용
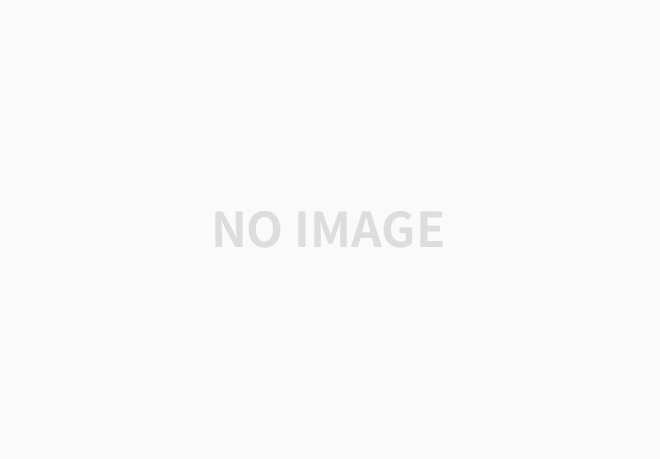
- Array, Dictionary, Set 타입에 사용
let arr = ["abcdef", "12345", "문자열"]
let bool = arr.allSatisfy { $0.count > 2 }
print(bool) // true
let dict = ["1": 1, "2": 2]
let bool2 = dict.allSatisfy { $0.key == String($0.value) }
print(bool2) // true
var set = Set<Int>()
set.insert(2)
set.insert(4)
set.insert(6)
let bool3 = set.allSatisfy { $0.isMultiple(of: 2) }
print(bool3) // true
Collection이란?
- Array, Set, Dictionary들이 모두 구현하고 있는 프로토콜
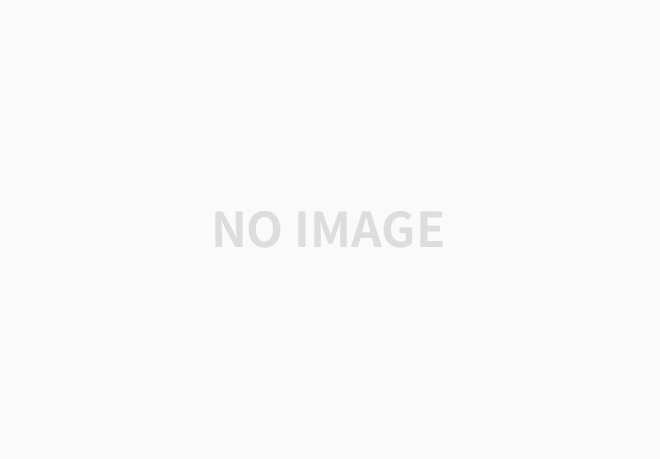
cf) Collection은 Sequence를 상속받고 있는 상태

- Sequence는 iterated하게 접근할 수 있는 elements를 가지고 있는 프로토콜

- Sequence는 Element와 Iterator 타입을 가지고 있는 형태
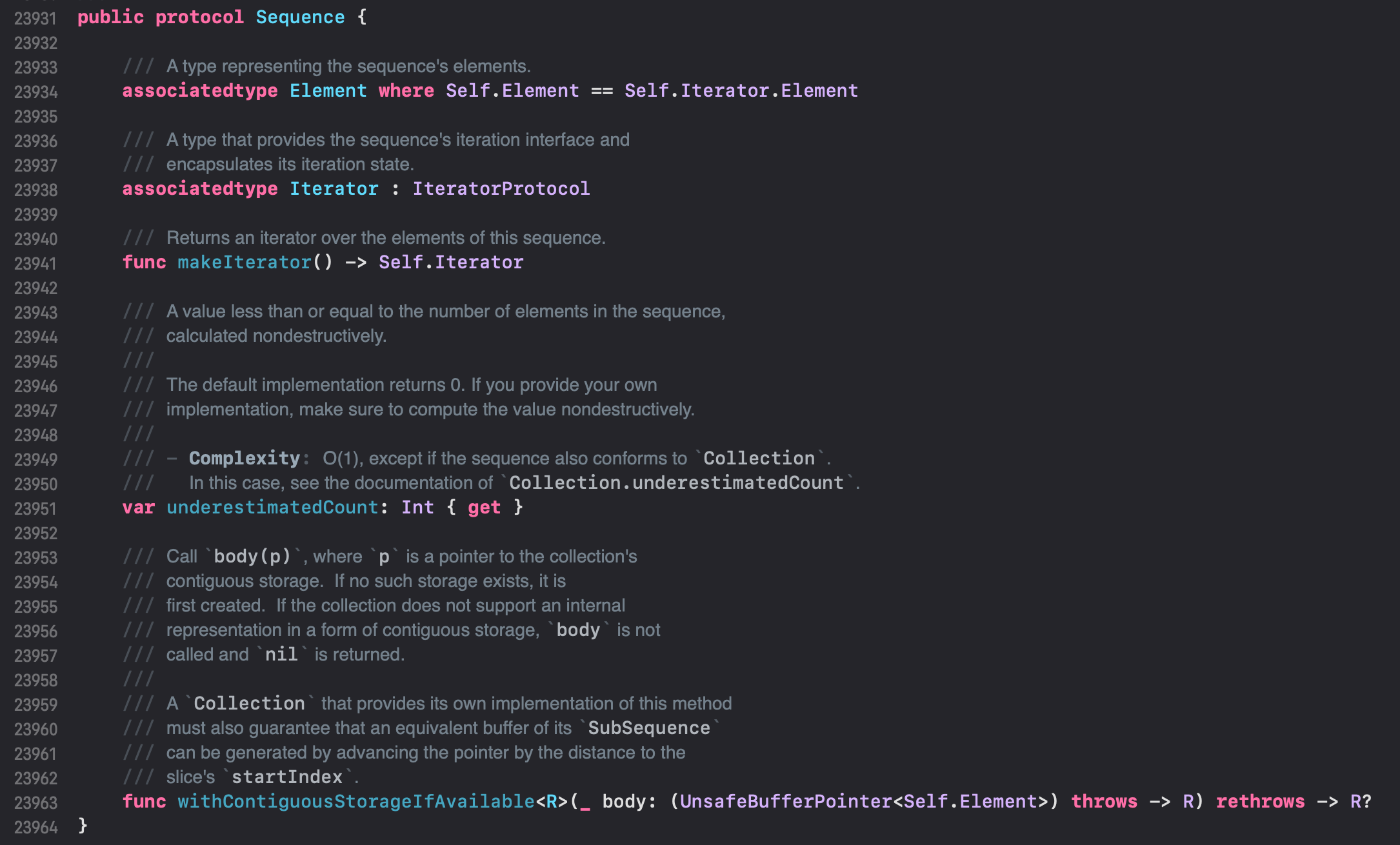
allSatisfy 연산자 응용 - contains
- 배열에 특정 값이 있는지 확인할 때 contains()를 아래처럼 사용하는데,
let arr = [1, 2, 3, 4, 5]
let element = 2
let result = arr.contains(element)
print(result) // true
- 배열에 특정 배열요소가 다 속하는지 확인할 때는 아래처럼 할 수 있지만,
let arr = [1, 2, 3, 4, 5]
let elements = [3, 4]
let result = arr.contains(all: elements)
print(result) // true
extension Array where Element: Equatable {
func contains(all elements: [Element]) -> Bool {
for element in elements where self.contains(element) == false {
return false
}
return true
}
}
- 더 간결한 방법은 allSatisfy를 사용
extension Array where Element: Equatable {
func contains(all elements: [Element]) -> Bool {
elements.allSatisfy(self.contains(_:))
}
}
* 참고
https://developer.apple.com/documentation/swift/array/2994715-allsatisfy
'iOS 기본 (swift)' 카테고리의 다른 글
Comments