Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- RxCocoa
- collectionview
- UITextView
- 애니메이션
- HIG
- Human interface guide
- scrollview
- 스위프트
- uiscrollview
- ios
- tableView
- UICollectionView
- ribs
- swiftUI
- map
- 클린 코드
- rxswift
- clean architecture
- Clean Code
- SWIFT
- swift documentation
- 리팩토링
- Observable
- 리펙토링
- uitableview
- Xcode
- Refactoring
- combine
- Protocol
- MVVM
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] 1. Local Notification (로컬 푸시, 로컬 노티) - 사용 방법 본문
iOS 응용 (swift)
[iOS - swift] 1. Local Notification (로컬 푸시, 로컬 노티) - 사용 방법
jake-kim 2022. 5. 7. 12:201. Local Notification (로컬 푸시, 로컬 노티) - 사용 방법
2. Local Notification (로컬 푸시, 로컬 노티) - badge (뱃지) 숫자 처리, 딥링크 처리
권한 요청
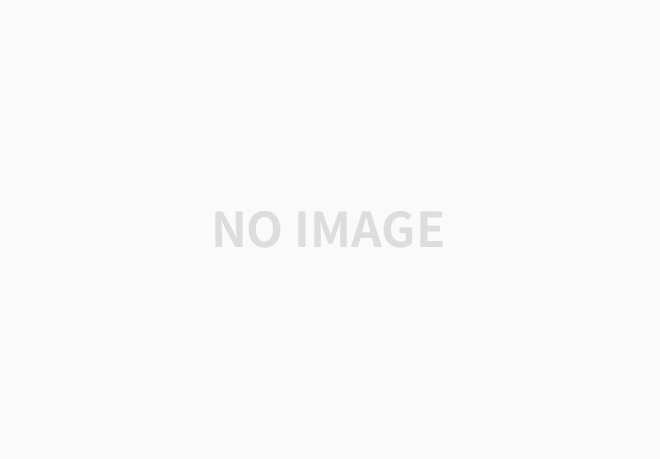
- 시스템 권한은 보통 info.plist에 하지만, 푸시는 특별하게 코드에서 요청
- UNUserNoitificationCenter.current() 싱글톤으로 접근
import UIKit
import UserNotifications
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
UNUserNotificationCenter.current().requestAuthorization(
// alert - 알림이 화면에 노출
// sound - 소리
// badge - 빨간색 동그라미 숫자
options: [.alert, .sound, .badge],
completionHandler: { (granted, error) in
print("granted notification, \(granted)")
}
)
return true
}
노티 보내기
- 노티 보내기 역시도 UNUserNotificationCenter.current() 사용
- 이 인스턴스의 add(_:) 메소드에 UNNotificationRequest를 넘기면 완료
- request정보에는 id, content, trigger 존재
@objc private func requestNoti() {
let content = UNMutableNotificationContent()
content.title = "노티 (타이틀)"
content.body = "노티 (바디)"
content.sound = .default
content.badge = 2
let request = UNNotificationRequest(
identifier: "local noti",
content: content,
trigger: UNTimeIntervalNotificationTrigger(
timeInterval: 3,
repeats: false
)
)
UNUserNotificationCenter.current()
.add(request) { error in
guard let error = error else { return }
print(error.localizedDescription)
}
}
- 테스트
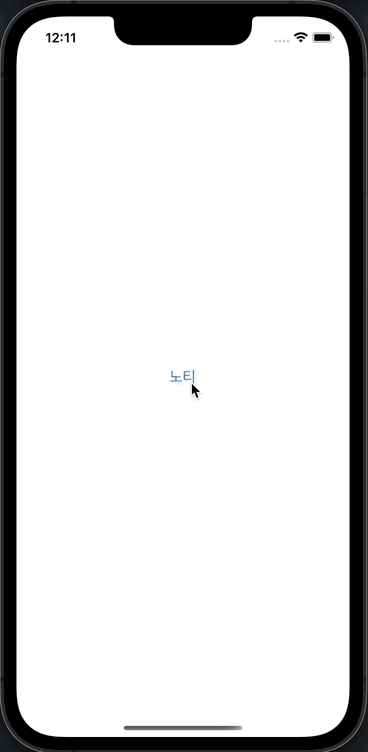
- 단, foreground에서도 푸시를 받고싶을때, AppDelegate에서 노티에 관한 delegate를 따로 처리가 필요
- 이 처리를 안해주면 background에서만 노티가 수신
foreground 에서도 노티 수신 방법
- application(_:didFinishLaunchingWithOptions:) 안에서 델리게이트 할당
UNUserNotificationCenter.current().delegate = self
- 델리게이트
- 옵션 list: 알림 센터에 표시 (상단에서 아래로 내리면 보이는 메뉴)
- 옵션 banner (화면 상단 알림)
extension AppDelegate: UNUserNotificationCenterDelegate {
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification) async -> UNNotificationPresentationOptions {
[.list, .banner, .sound, .badge]
}
}
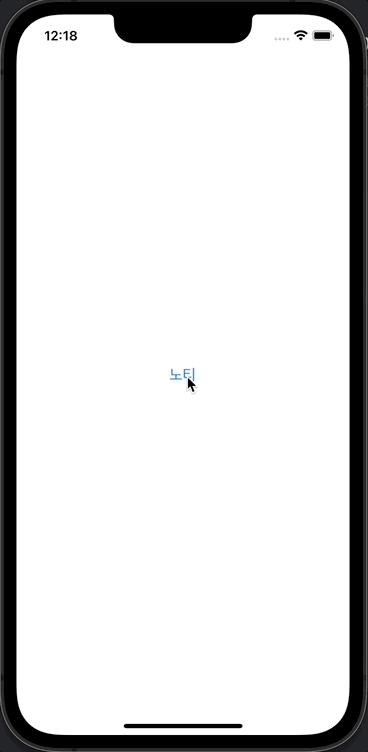
* 전체 코드: https://github.com/JK0369/ExLocalPush1
* 참고
'iOS 응용 (swift)' 카테고리의 다른 글
[iOS - swift] Enum에서 초기화하는 테크닉 (~= 연산자) (0) | 2022.05.09 |
---|---|
[iOS - swift] 2. Local Notification (로컬 푸시, 로컬 노티) - badge (뱃지) 숫자 처리, 딥링크 처리 (0) | 2022.05.08 |
[iOS - swift] 2. UISegmentedControl - 커스텀 방법, PageViewController와 사용 방법 (3) | 2022.05.06 |
[iOS - swift] 1. UISegmentedControl - 기본 사용 방법 (0) | 2022.05.05 |
[iOS - swift] 별점 팝업, 리뷰 요청 팝업 띄우는 방법 StoreKit, SKStoreReviewController (0) | 2022.05.04 |