일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
- Human interface guide
- collectionview
- map
- 리펙토링
- SWIFT
- 스위프트
- ribs
- Clean Code
- clean architecture
- ios
- HIG
- tableView
- UICollectionView
- MVVM
- uitableview
- 리펙터링
- uiscrollview
- RxCocoa
- 애니메이션
- rxswift
- Xcode
- Observable
- swift documentation
- 클린 코드
- Protocol
- Refactoring
- UITextView
- combine
- swiftUI
- 리팩토링
- Today
- Total
목록swift 공식 문서 (27)
김종권의 iOS 앱 개발 알아가기
Method 메서드의 정의: 특정 유형과 관련된 함수 인스턴스 작업을 위한 특정 작업 Instance Method 특정 클래스, 구조, 열거 형의 인스턴스에 속하는 함수 class Counter { var count = 0 func increment() { count += 1 } func increment(by amount: Int) { count += amount } func reset() { count = 0 } } let counter = Counter() // 1 counter.increment() // 1 counter.increment(by: 5) // 6 counter.reset() // 0 Self Property 클래스 내에서 self의 의미: 자체 인스턴스 메서드 내에서 현재 인스턴스를..
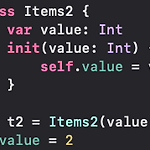
Properties 내부에 property가 var로 선언되어도, copy of value인 struct는 인스턴스를 let으로 할 경우 변경 불가 내부에 property가 var로 선언되고, reference of value인 class는 인스턴스를 let으로 할 경우 변경 가능 Lazy stored property 상수 속성은 초기화가 완료되기 전에 항상 값을 가져야 하므로 lazy 선언 불가 ex) 불필요한 초기화를 피하기 위해 lazy stored property 사용 class DataImporter { var filename = "data.txt" } class DataManager { lazy var importer = DataImporter() var data: [String] = [] }..
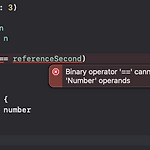
Structures and Classes swift에서는 class의 인스턴스를 object라고 하지 않고, 기능에 가까운 언어이므로 "instance"라고 명명 Class에서만 있는 속성 상속 type casting: 런타임에 클래스 instance의 유형을 확인하고 해석 Deinitialzer는 클래스의 instance가 할당 된 리소스를 해제할 수 있도록 하는 기능 reference counting을 통해 한 클래스 instance에 대한 하나 이상의 참조를 허용 Structure와 Enum은 value type copy - by - value let hd = Resolution(width: 1920, height: 1080) var cinema = hd cinema.width = 2048 // c..
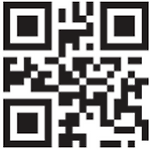
Enumerations enum은 value type Enum 네이밍: 복수가 아닌 단수형태이고 대문자로 시작 enum CompassPoint { case north case south case east case west } var directionToHead = CompassPoint.west CaseIterable case들의 사례를 모두 가져오고 싶은 경우 CaseIterable을 conform하여 구현 'allCases' 프로퍼티 사용 enum Beverage: CaseIterable { case coffee, tea, juice } let numberOfChoices = Beverage.allCases.count print("\(numberOfChoices) beverages available"..
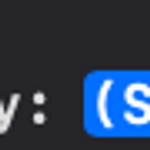
Closure Closure(폐쇄): 클로저는 코드에서 전달 및 사용할 수 있는 2가지의 자체 기능을 가진 블록 정의된 컨텍스트에서 모든 상수 및 변수에 대한 참조를 캡쳐하고 저장 가능 캡쳐: 자신의 블록 외부에 있는 값을 참조하는 것 // ex) 캡쳐: incrementer() 함수 블록에서 runningTotal과 amount인수를 참조 func makeIncrementer(forIncrement amount: Int) -> () -> Int { var runningTotal = 0 func incrementer() -> Int { runningTotal += amount return runningTotal } return incrementer } Closure는 실행 순서 제어가능 클로저는 호출할 ..
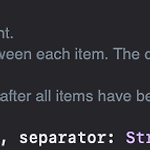
print(_:separator:terminator) separator: 각 item 사이의 공간 space (디폴트 " ") terminator: print안의 아이템들을 모두 출력 후 마지막 문자 (디폴트 '\n') parameter에 함수 유형 고차 함수(higher order function) 성격: 함수를 매개변수, 변수로 받을 수 있고 리턴 할 수 있는 성질 cf) 일급 객체 성격: 함수를 변수에 대입할 수 있는 형태 higher order function의 개념: https://ios-development.tistory.com/104 ex) 함수를 매개변수로 받는 형태 func printMathResult(_ mathFunction: (Int, Int) -> Int, _ a: Int, _ b..
For - in 루프 stride(from:to:by:) stride(from:through:by:): through 포함 While 루프 repeat - while: 조건을 고려하기 전 repeat블록을 부조건 한번 실행 repeat { } while 조건문 switch 문의 암시적 fall through case문을 여러개 사용하지 않고, 컴마로 구분하여 'or 조건' 사용 (주의: if문에서는 콤마가 'end 조건') let anotherCharacter: Character = "a" // 컴파일 에러 switch anotherCharacter { case "a": case "A": print("The letter A") default: print("Not the letter A") } // 정상 ..
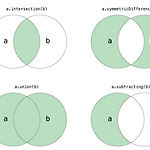
Swift 컬렉션은 3가지 Array Set Dictionary Array 갯수와 값을 지정한 초기화 let intArr = [Int](repeating: 0, count: 10) 추가: '+=' 연산자 사용 intArr += [2] 삽입: insert(_:at:) 기존에 at에 있던 값은 오른쪽으로 밀려나는 형태 var intArr = [Int](repeating: 0, count: 3) // [0, 0, 0] intArr.insert(2, at: 1) // [0, 2, 0, 0] 삭제: remove(at:) var intArr = [Int](repeating: 0, count: 3) // [0, 0, 0] intArr.insert(2, at: 1) // [0, 2, 0, 0] let removedVa..