일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- Clean Code
- combine
- map
- 리팩토링
- Protocol
- SWIFT
- swift documentation
- ios
- uitableview
- Observable
- clean architecture
- tableView
- Refactoring
- Xcode
- uiscrollview
- RxCocoa
- 리펙토링
- HIG
- MVVM
- UITextView
- UICollectionView
- 스위프트
- swiftUI
- collectionview
- rxswift
- 애니메이션
- 클린 코드
- Human interface guide
- scrollview
- ribs
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - UI Custom] 7. 커스텀 클래스 - UIButton 본문
[iOS - UI Custom] 7. 커스텀 클래스 - UIButton
jake-kim 2020. 4. 17. 20:31
- 특정 클래스를 상속받아서(UIButton, UILabel,,,) UI를 구현
- 완성된 커스텀 클래스는 객체를 생성하여 바로 사용 & storyboard에서 클래스 연결하여 사용
*커스텀 클래스에서 중요한 기본내용은 init개념
[swift] 13. 초기화(init, convenience init, required init, override init)
let b = Boo() 원래의 의미는 "let b = Boo.init()" 1. init의 존재 이유 - 모든 저장 프로퍼티들은 초기화 되어야 함, init키워드는 이것을 도와줌 1 2 3 4 5 6 7 8 9 10 11 class Test{ var a:Int! // nil로 초..
ios-development.tistory.com
1. UIButton 커스텀 기본 구성
1) 세 가지 기본 init 구현
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
class CSButton: UIButton {
// 스토리보드에서 참고
required init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)!
}
override init(frame: CGRect){
super.init(frame: frame)
}
init() {
super.init(frame:CGRect.zero)
}
}
|
2) 커스텀정의 : 적절한 초기화 구문에 "self."로 접근하여 속성 지정
1
2
3
4
5
6
7
8
9
10
11
|
// CSButton.swift
init() {
super.init(frame:CGRect.zero)
self.backgroundColor = .black
self.layer.borderColor = UIColor.black.cgColor
self.layer.borderWidth = 2
self.layer.cornerRadius = 2
self.setTitleColor(.white, for: .normal)
self.setTitle("custom button", for: .normal)
}
|
3) 커스텀 사용
1
2
3
4
5
6
7
|
// ViewController.swift
override func viewDidLoad() {
super.viewDidLoad()
let btn = CSButton()
btn.frame = CGRect(x: 50, y: 100, width: 150, height: 30)
self.view.addSubview(btn)
}
|
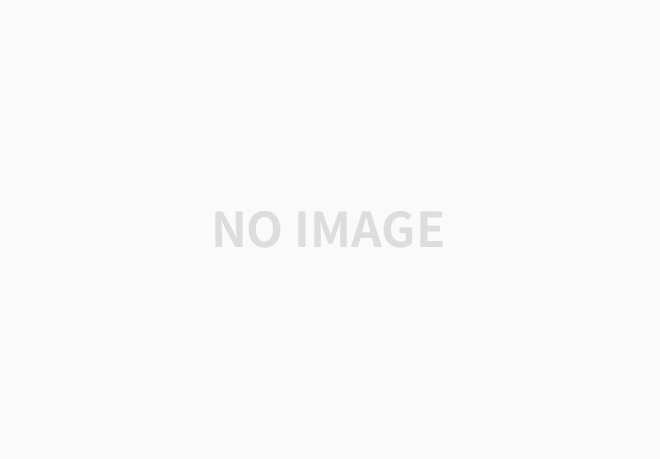
2. 편리하게 다룰 수 있도록 Custom하기
1) enum상수 이용 : 외부에서 ".black"과 같이 쉽게 이용 가능
1
2
3
4
5
6
7
|
// CSButton.swift
// enum사용시, 외부에서도 ".rect"와 같이 쉽게 접근 할 수 있음
public enum CSButtonType {
case rect
case circle
}
|
|
2) 객체 생성시, 스타일을 선택할 수 있도록 convenience init 이용
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
// CSButton.swift
init() {
super.init(frame:CGRect.zero)
}
// 초기화 시, 타입 지정 가능 : "var btn = CSButton(type: .rect)"
convenience init(type: CSButtonType) {
self.init()
switch type {
case .rect:
self.backgroundColor = .black
self.layer.borderColor = UIColor.black.cgColor
self.layer.borderWidth = 2
self.layer.cornerRadius = 0
self.setTitleColor(.white, for: .normal)
self.setTitle("Rect button", for: .normal)
case .circle:
self.backgroundColor = .red
self.layer.borderColor = UIColor.blue.cgColor
self.layer.borderWidth = 2
self.layer.cornerRadius = 50
self.setTitle("Circle Button", for: .normal)
}
}
|
3) 객체생성 후 프로퍼티로 접근할 수 있도록 연산프로퍼티(didset)정의
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
// didSet 연산 프로퍼티 정의하면, 외부에서 "객체.style ="꼴로 접근하기 쉬움
var style: CSButtonType = .rect {
didSet {
switch style {
case .rect:
self.backgroundColor = .black
self.layer.borderColor = UIColor.black.cgColor
self.layer.borderWidth = 2
self.layer.cornerRadius = 0
self.setTitleColor(.white, for: .normal)
self.setTitle("Rect button", for: .normal)
case .circle:
self.backgroundColor = .red
self.layer.borderColor = UIColor.blue.cgColor
self.layer.borderWidth = 2
self.layer.cornerRadius = 50
self.setTitle("Circle Button", for: .normal)
}
}
}
|
- 사용
1
2
3
4
5
6
7
8
9
|
// ViewController.swift
override func viewDidLoad() {
super.viewDidLoad()
let btn = CSButton(type: .circle)
btn.frame = CGRect(x: 50, y: 100, width: 150, height: 30)
btn.style = .rect
self.view.addSubview(btn)
}
|
3. Action메소드 삽입
1) @objc func 메소드 정의
1
2
3
4
|
// CSButton.swift
@objc func changeColor(_ sender: UIButton) {
self.backgroundColor = .cyan
}
|
2) self.addTarget(self, action:, for:)로 정의
1
2
3
4
5
6
7
8
|
// CSButton.swift
convenience init(type: CSButtonType) {
self.init()
(중략)
self.addTarget(self, action: #selector(changeColor(_:)), for: .touchUpInside)
}
|
* 이벤트를 부르는 메소드 : sendAction(for:)
[iOS - UI Custom] 9. 커스텀 클래스 - UIStepper
* 형태 - UIControl을 상속 받아서, 그곳에 UIButton 두 개와, UILabel하나 추가 - (UIControl과 UIView 둘 중 하나를 상속 받아도 가능하지만 UIControl을 상속받으면 객체에 이벤트 리스너(UIControl.event) 등..
ios-development.tistory.com
'iOS 실전 (swift) > UI 커스텀(프로그래밍적 접근)' 카테고리의 다른 글
[iOS - UI Custom] 9. 커스텀 클래스(UIControl상속) - UIStepper (0) | 2020.04.18 |
---|---|
[iOS - UI Custom] 8. 커스텀 클래스 - UITabBarController (0) | 2020.04.18 |
[iOS - UI Custom] 6. 경고창(UIAlertController) (0) | 2020.04.17 |
[iOS - UI Custom] 5. TabBar Controller (0) | 2020.04.17 |
[iOS - UI Custom] 4. Navigation Controller (0) | 2020.04.17 |
jake-kim님의
글이 좋았다면 응원을 보내주세요!