Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |
Tags
- RxCocoa
- MVVM
- 리팩토링
- SWIFT
- uiscrollview
- collectionview
- HIG
- Clean Code
- Observable
- 스위프트
- UITextView
- Human interface guide
- map
- tableView
- Refactoring
- Xcode
- scrollview
- 애니메이션
- Protocol
- ios
- UICollectionView
- rxswift
- ribs
- combine
- swiftUI
- clean architecture
- uitableview
- 클린 코드
- swift documentation
- 리펙토링
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] ProgressBar 구현 방법 본문
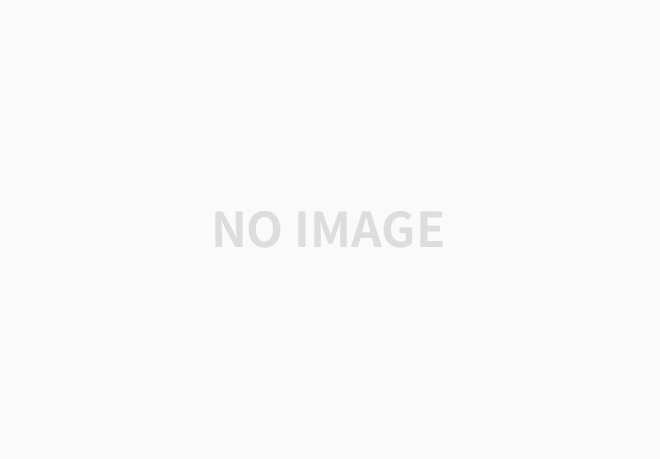
* 구현에서 편리한 레이아웃 작성을 위해 사용한 프레임 워크
pod 'SnapKit'
구현 아이디어
- AutoLayout을 이용하여 progressBar의 width를 업데이트하면서 progressBar 구현
- progress를 업데이트하면 로딩이 매끄럽지 않고 끊겨져 보일 수 있으므로, UIView.animate 사용
구현
- ProgressBarView 정의
import UIKit
import SnapKit
final class ProgressBarView: UIView {
}
- progressBarView 뷰 정의
private let progressBarView: UIView = {
let view = UIView()
view.backgroundColor = .systemBlue
return view
}()
- 초기화 구문
- isUserInteractionEnabled를 false로 설정하여 interaction 비활성화
- backgroundColor 설정하여 배경 색상 정의
override init(frame: CGRect) {
super.init(frame: frame)
self.isUserInteractionEnabled = false
self.backgroundColor = .systemGray
self.addSubview(self.progressBarView)
}
required init?(coder: NSCoder) {
fatalError()
}
- ratio 프로퍼티를 두어, didSet에서 progress 처리
- width값을 equalToSuperview().multipliedBy()를 통해 progress 게이지 업데이트
- UIView.animate()를 통해 게이지가 업데이트 될 때 매끄럽게 되도록 구현
var ratio: CGFloat = 0.0 {
didSet {
self.isHidden = !self.ratio.isLess(than: 1.0)
self.progressBarView.snp.remakeConstraints {
$0.top.bottom.equalTo(self.safeAreaLayoutGuide)
$0.width.equalToSuperview().multipliedBy(self.ratio)
}
UIView.animate(
withDuration: 0.5,
delay: 0,
options: .curveEaseInOut, // In과 Out 부분을 천천히하라는 의미 (나머지인 중간 부분은 빠르게 진행)
animations: self.layoutIfNeeded, // autolayout에 애니메이션을 적용시키고 싶은 경우 사용
completion: nil
)
}
}
사용하는 쪽
import UIKit
import SnapKit
class ViewController: UIViewController {
private let progressBarView = ProgressBarView()
override func viewDidLoad() {
super.viewDidLoad()
self.title = "ProgressBar 테스트"
self.view.addSubview(self.progressBarView)
self.progressBarView.snp.makeConstraints {
$0.left.top.right.equalTo(self.view.safeAreaLayoutGuide)
$0.height.equalTo(5)
}
var timer = 0.0
let finish = 10.0
Timer.scheduledTimer(
withTimeInterval: 1,
repeats: true) { _ in
timer += 1
self.progressBarView.ratio = timer / finish
}
}
}