Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- HIG
- Protocol
- ios
- ribs
- 애니메이션
- RxCocoa
- uitableview
- 스위프트
- swiftUI
- map
- rxswift
- SWIFT
- 클린 코드
- Human interface guide
- swift documentation
- uiscrollview
- UITextView
- Refactoring
- tableView
- collectionview
- Xcode
- scrollview
- 리팩토링
- combine
- UICollectionView
- Clean Code
- MVVM
- Observable
- clean architecture
- 리펙토링
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] 1. Diffable Data Source - UITableViewDiffableDataSource (테이블 뷰) 본문
iOS 응용 (swift)
[iOS - swift] 1. Diffable Data Source - UITableViewDiffableDataSource (테이블 뷰)
jake-kim 2021. 10. 2. 22:301. Diffable Data Source - UITableViewDiffableDataSource (테이블 뷰)
2. Diffable Data Source - UICollectionViewDiffableDataSource (컬렉션 뷰)
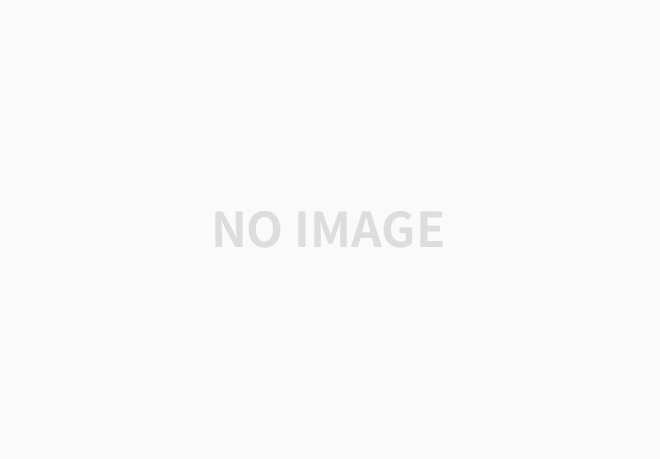
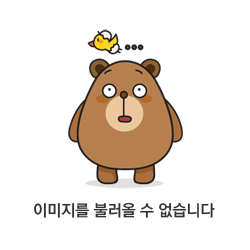
원리
- 기존 Controller와 UI의 관계
- UI가 Controller에게 cell의 모양(cellForRowAt), cell의 개수(numberOfRowsInSection)을 델리게이트로부터 받아서 처리
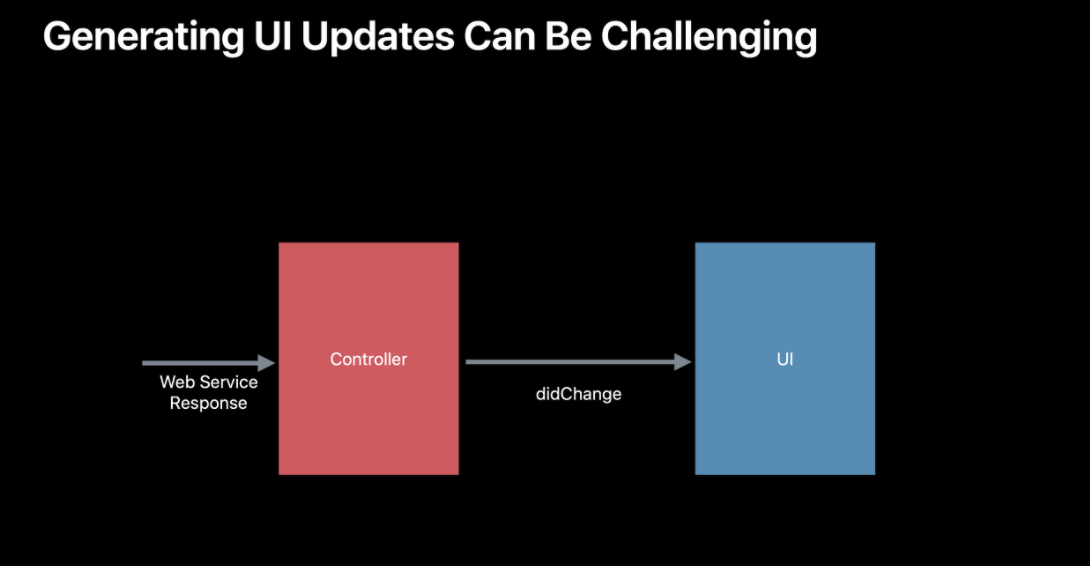
- 기존은 특정 cell만 바뀐경우의 처리는 아래와 같이 처리
- 방법 1) performBatchUpdates()의 클로저 블록에 데이터 변경 작업 추가
- 방법 2) beginUpdates()와 endupdates() 사이에 데이터 변경 작업 작성
- iOS13+에서 단순히 apply()를 통해 위 작업들을 처리 가능
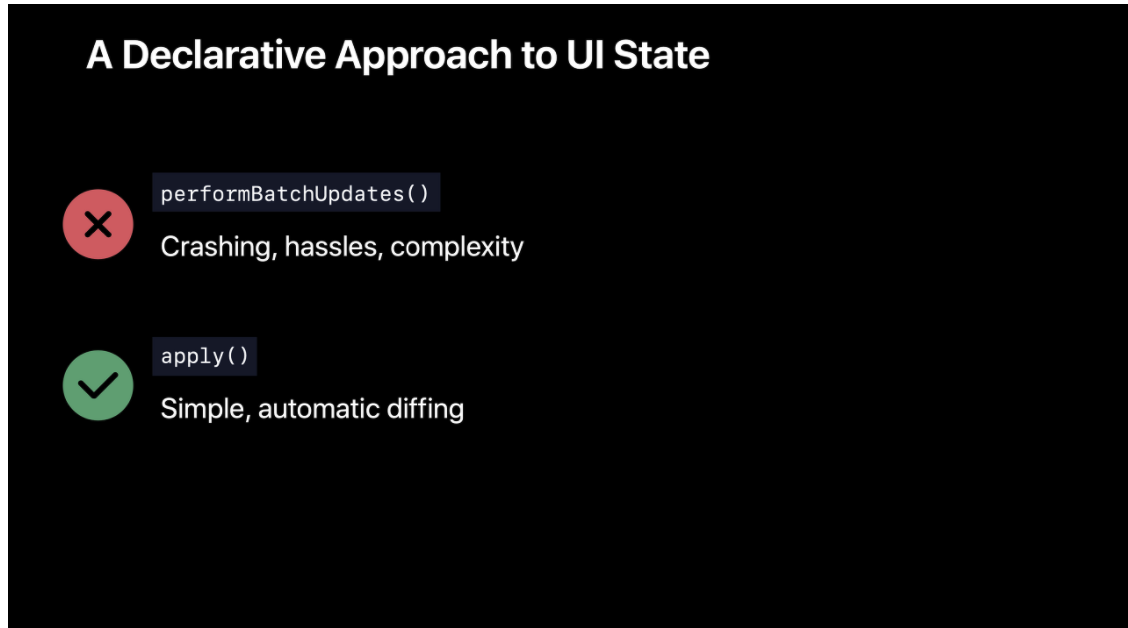
- UITableViewDiffableDataSource는 IndexPath를 사용하지 않고 Hash개념을 사용
- 빠른 속도 보장
- 간결성
- IndexPath를 잘못 입력하여 크래시날 확률이 줄어드는 장점도 존재
- 자동으로 cell이 사라지고 생기는 애니메이션 동작
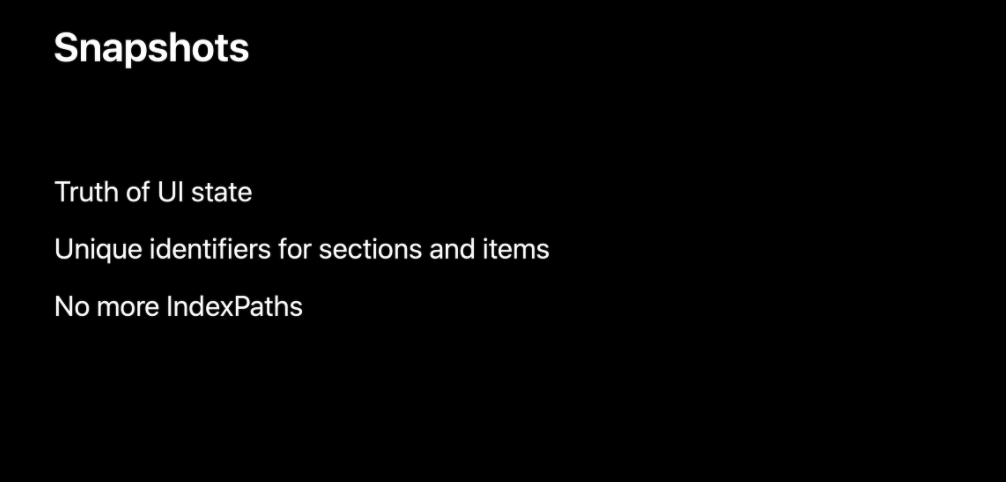
사용 방법
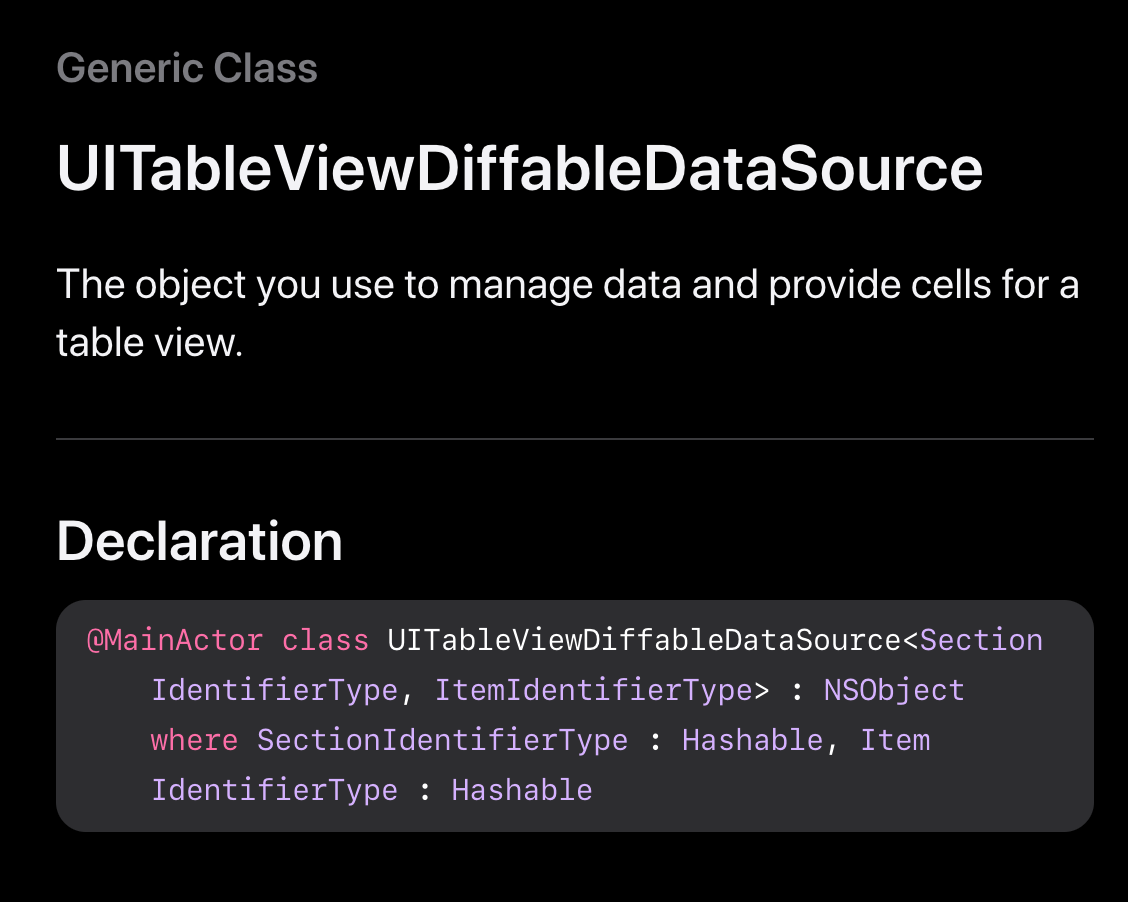
- UITableViewDiffableDataSource<SectionIdentifierType, ItemIdentifierType> 정의
- SectionIdentifierType: 보통 enum: Int, CaseIteerable을 준수하는 enum으로 정의
- ItemIdentifierType: Hashable을 따르는 Cell 타입
class ViewController: UIViewController {
var dataSource: UITableViewDiffableDataSource<Int, UUID>!
}
- tableView에 cell 등록
tableView.register(UITableViewCell.self, forCellReuseIdentifier: "myCell")
- cell의 모양 정의 (기존에 사용하던 cellForRowAt 델리게이트 메소드 역할)
dataSource = UITableViewDiffableDataSource<Int, UUID>(tableView: tableView, cellProvider: { tableView, indexPath, itemIdentifier in
let cell = tableView.dequeueReusableCell(withIdentifier: "myCell", for: indexPath)
cell.backgroundColor = self.colors[indexPath.row % self.colors.count]
return cell
})
- tableView의 dataSource프로퍼티에 위 프로퍼티를 대입
tableView.dataSource = dataSource
- tableView에 들어갈 초기값 세팅
- snapshot의 의미: 현재 UI의 상태를 의미하고 여기에 appendSections, appendItems 후에 이 snapshot을 tableView에 대입되어 있는 dataSource객체에 apply 파라미터로 넣어주면 자동으로 셀 업데이트
var snapshot = NSDiffableDataSourceSnapshot<Int, UUID>()
snapshot.appendSections([0]) // 주의: section하나를 안넣어주면 에러
snapshot.appendItems([UUID(), UUID(), UUID()])
dataSource.apply(snapshot)
데이터 append, insert, delete
@IBAction func didTapAppendButton(_ sender: Any) {
var snapshot = dataSource.snapshot()
snapshot.appendItems([UUID()])
dataSource.apply(snapshot)
}
// 첫번째 item에 삽입
@IBAction func didTapInsertButton(_ sender: Any) {
var snapshot = dataSource.snapshot()
if let first = snapshot.itemIdentifiers.first {
snapshot.insertItems([UUID()], afterItem: first)
}
dataSource.apply(snapshot)
}
// 마지막 item 삭제
@IBAction func didTapDeleteButton(_ sender: Any) {
var snapshot = dataSource.snapshot()
if let lastItem = snapshot.itemIdentifiers.last {
snapshot.deleteItems([lastItem])
}
dataSource.apply(snapshot)
}
* Diffable Data Source 응용 - MVVM에서 사용 방법은 이 포스팅 글에서 확인
* 전체 소스코드
- ViewController 클래스 내용: https://github.com/JK0369/Ex_UITableViewDiffableDataSource
* 참고
- UITableViewDiffableDataSource<SectionIdentifierType, ItemIdentifierType>:
https://developer.apple.com/documentation/uikit/uitableviewdiffabledatasource