Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- HIG
- map
- uitableview
- Xcode
- collectionview
- 애니메이션
- ribs
- UICollectionView
- combine
- ios
- SWIFT
- tableView
- scrollview
- 클린 코드
- clean architecture
- Clean Code
- 리팩토링
- Refactoring
- Observable
- MVVM
- Protocol
- rxswift
- 스위프트
- 리펙토링
- Human interface guide
- swiftUI
- RxCocoa
- UITextView
- uiscrollview
- swift documentation
Archives
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] ARC, strong, unowned, weak (실무 관점) 본문
ARC
- Auto Reference Count
- block에 있는 프로퍼티들에 대하여 RC(Reference count)를 0으로 만들기 위해 -1씩 RC를 감소시켜 메모리해제를 자동으로 해주는 것
- 단 retain cycle인 경우 메모리 해제 불가능한 경우 존재
- 주의: 특정 블럭의 함수가 종료된 경우에 메모리 해제를 진행하는 것
- weak는 RC를 늘리지 않아서 RC가 0으로 유지되지만 0이라고 곧바로 메모리 해제가 되는것이 아닌 것
Strong
- RC(Reference Count)를 증가
class A {
var b: B?
}
class B {
var a: A?
}
func sumFunction() {
var aInstanceReference: A? = A() // a_RC = 1
var bInstanceReference: B? = B() // b_RC = 1
aInstanceReference?.b = bInstanceReference // b_RC = 2
}
- a객체의 참조 카운트는 1, b객체의 참조 카운트는 2인 상태
- sumFuntion 함수가 종료되면 a객체 b객체 모두 참조카운트가 -1씩 감소
- a 객체의 참조 카운트 = 0
- b 객체의 참조 카운트 = 1 -> b객체는 현재 a객체를 참조하고 있는데 a객체의 RC값이 0이 되었으므로, b객체의 참조 카운트는 0으로 변경
- a, b객체 모두 힙에서 메모리 해제
- Retain Cycle
func sumFunction() {
var aInstanceReference: A? = A() // a_RC = 1
var bInstanceReference: B? = B() // b_RC = 1
aInstanceReference?.b = bInstanceReference // b_RC = 2
bInstanceReference?.a = aInstanceReference // a_RC = 2
}
- a객체 b객체 모두 참조 카운트 2인 상태
- sumFunction함수가 종료되면 a객체 b객체 모두 참조카운트가 -1씩 감소
- a 객체의 참조 카운트 = 1
- b 객체의 참조 카운트 = 1
- 함수가 종료되어도 block안의 지역변수들이 힙에서 메모리 해제가 안된 상태
Retain cycle 그림
var aInstanceReference: A? = A()
var bInstanceReference: B? = B()
aInstanceReference?.b = bInstanceReference
bInstanceReference?.a = aInstanceReference
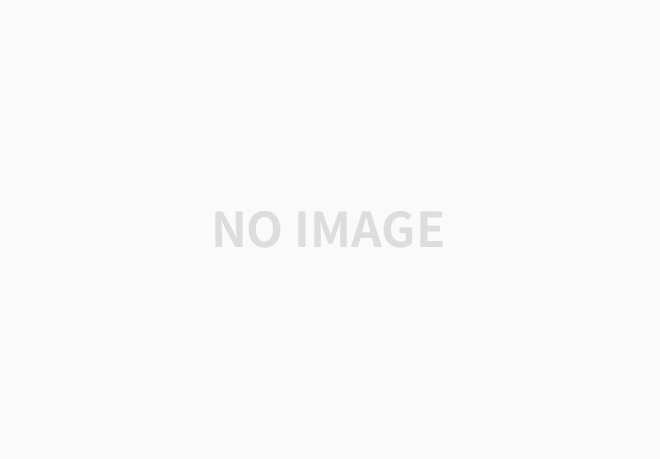
aInstanceReference = nil
bInstanceReference = nil
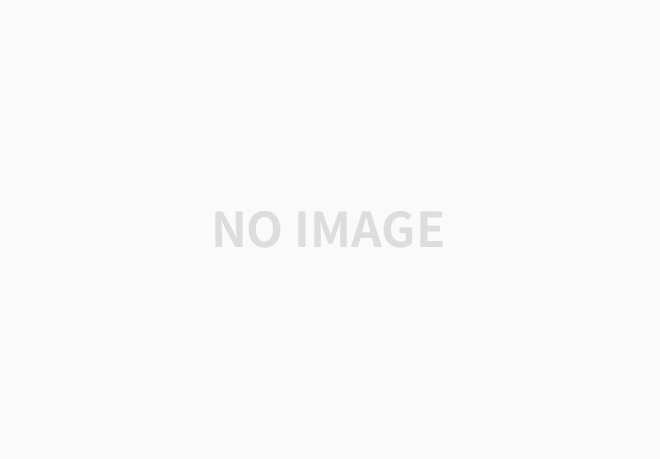
weak
- RC값을 늘려주지 않는 형태
- weak를 사용할땐 반드시 Optional 타입 (해당 객체가 nil이 될 수 있기 때문)
- 객체를 참조하다가, 객체가 사라지면 nil을 가리키는 형태
unowned
- RC값을 늘려주지 않는 형태
- 객체를 참조하다가, 객체가 사라지면 nil을 가리킬 수 없으므로 댕글링 포인터(Dangling Pointer)를 바라보며 에러가 발생
* Memory leak 확인 방법: https://ios-development.tistory.com/604
'iOS 기본 (swift)' 카테고리의 다른 글
[iOS - swift] SPM(Swift Pacakage Manager) 사용 방법 (0) | 2021.05.22 |
---|---|
[iOS - swift] xib 사용원리 (archive, unarchive), encode, decode, NSCoder (0) | 2021.05.19 |
[iOS - swift] weak var 사용방법 ('weak' must not be applied to non-class-bound) (0) | 2021.04.29 |
[iOS - swift] ~= 연산자 (범위 연산자) (0) | 2021.04.08 |
[iOS - swift] Argument Labels vs Parameter (0) | 2021.04.08 |