일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- Clean Code
- RxCocoa
- HIG
- clean architecture
- 애니메이션
- swift documentation
- UICollectionView
- combine
- tableView
- 클린 코드
- Xcode
- MVVM
- scrollview
- map
- swiftUI
- uitableview
- 스위프트
- 리팩토링
- SWIFT
- collectionview
- Human interface guide
- UITextView
- Observable
- Protocol
- 리펙토링
- ios
- Refactoring
- ribs
- rxswift
- uiscrollview
- Today
- Total
김종권의 iOS 앱 개발 알아가기
[iOS - swift] 3. Push Notification 응용 - Rich Push Notification (Notification Service Extension, 푸시 내용 변경하여 띄우기) 본문
[iOS - swift] 3. Push Notification 응용 - Rich Push Notification (Notification Service Extension, 푸시 내용 변경하여 띄우기)
jake-kim 2023. 2. 19. 22:371. Push Notification 응용 - 테스트 방법 (Pusher, APNs)
2. Push Notification 응용 - Silent Push Notification (사일런트 푸시, 푸시를 이용한 백그라운드에서 업데이트 방법)
3. Push Notification 응용 - Rich Push Notification (Notification Service Extension, 푸시 내용 변경하여 띄우기) <
4. Push Notification 응용 - 시스템 푸시에 이미지 넣기 (Notification Service Extension, mutable-content)
5. Push Notification 응용 - 푸시 커스텀 UI 구현 방법 (Notification Content Extension, category)
6. Push Notification 응용 - 푸시 앱 아이콘 부분 커스텀 방법 (메시지 앱에서의 썸네일 아이콘 푸시 구현, 카톡 푸시 썸네일, INSendMessageIntent)
Rich Push Notification
- Push Notification의 payload를 그대로 띄워주는게 아닌, Intercept하여 푸시 내용의 payload를 가로채어 썸네일이나 특별한 작업을 수행
- Notification Service Extension을 사용하면 push notification이 띄워지기 전에 가로채어 특정 작업 수행이 가능
- 시스템 푸시에 썸네일이나 사진도 표현이 가능
푸시 환경 준비
- 이전 포스팅 글 1번글을 참고하여 푸시 테스트 환경 준비
- apple developer에서 keys 생성
- provisioning profile 생성
- Xcode push notification 활성화 (background modes, push notifications)
- Pusher로 테스트
Notification Service Extension으로 구현하기
- Xcode > New > Target
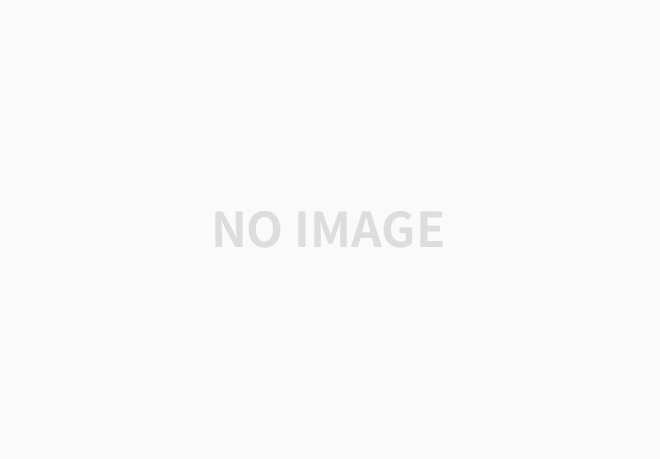
- Notification Service Extension 선택
- 옆에 Content Extension은 시스템 푸시의 UI를 변경할 때 사용
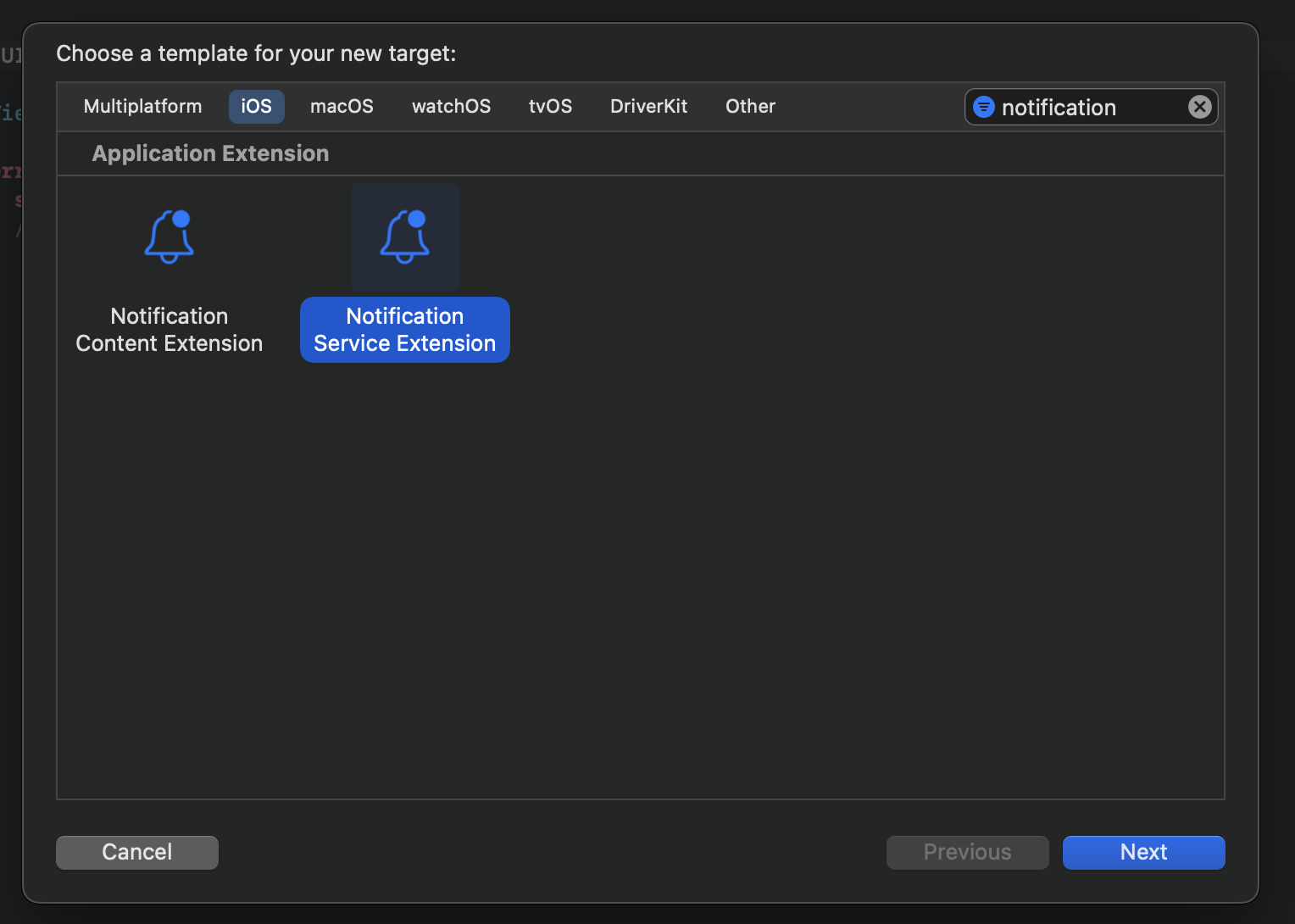
- 생성 완료
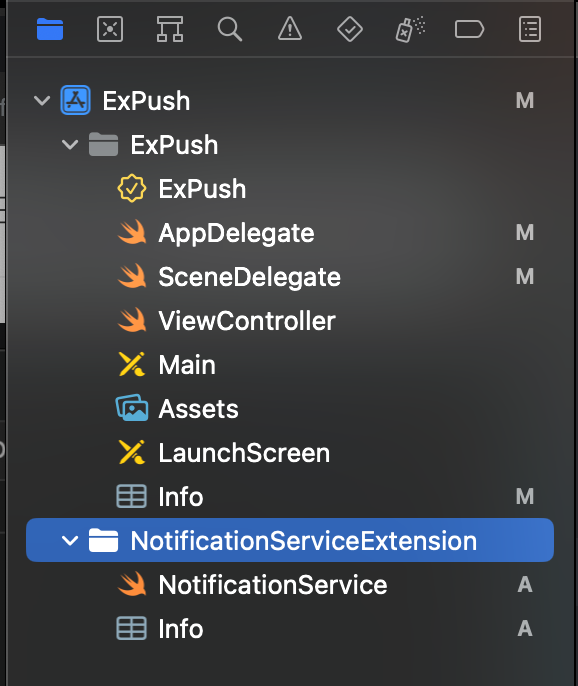
- NotificationService로 APNs 푸시 가로채서 title, subtitle 내용 변경하여 띄우기
- 위에서 만든 NotificationServiceExtension 코드
import UserNotifications
class NotificationService: UNNotificationServiceExtension {
var contentHandler: ((UNNotificationContent) -> Void)?
var bestAttemptContent: UNMutableNotificationContent?
// APNs를 수신하면 didReceive 메소드 호출
// contentHnadler 클로저를 수행하면 푸시가 노출
override func didReceive(_ request: UNNotificationRequest, withContentHandler contentHandler: @escaping (UNNotificationContent) -> Void) {
}
// didReceive에서 contentHandler가 호출되지 않고 특정 시간이 경과하면 이 메소드가 호출
override func serviceExtensionTimeWillExpire() {
if let contentHandler = contentHandler, let bestAttemptContent = bestAttemptContent {
contentHandler(bestAttemptContent)
}
}
}
- serviceExtensionTimeWillExpire()은 didReceive에서 handler를 처리하지 않은 경우 호출
- didReceive에서 APNs의 원본 데이터와 수정하여 띄우기가 가능
- contentHandler는 전역적으로 바로 저장 (didReceive에서 작업을 다 못한 경우 serviceExtensionTimeWillExpire 메소드에서 처리하기 위함)
- UNMutableNotificationContent 인스턴스 생성
// APNs를 수신하면 didReceive 메소드 호출
// contentHnadler 클로저를 수행하면 푸시가 노출
override func didReceive(_ request: UNNotificationRequest, withContentHandler contentHandler: @escaping (UNNotificationContent) -> Void) {
self.contentHandler = contentHandler
bestAttemptContent = (request.content.mutableCopy() as? UNMutableNotificationContent)
}
- UNMutableNotificationContent 이란?
- notification content의 정보를 가지고 있는 클래스
open class UNMutableNotificationContent : UNNotificationContent {
open var attachments: [UNNotificationAttachment]
@NSCopying open var badge: NSNumber?
open var body: String
open var categoryIdentifier: String
open var launchImageName: String
@NSCopying open var sound: UNNotificationSound?
open var subtitle: String
open var threadIdentifier: String
open var title: String
open var userInfo: [AnyHashable : Any]
open var summaryArgument: String
open var summaryArgumentCount: Int
open var targetContentIdentifier: String? // default nil
open var interruptionLevel: UNNotificationInterruptionLevel
open var relevanceScore: Double
open var filterCriteria: String? // default nil
}
- didReceive에서 위 인스턴스로 적절한 값을 세팅해주고 handler를 실행시키면 시스템 푸시에 반영
// APNs를 수신하면 didReceive 메소드 호출
// contentHnadler 클로저를 수행하면 푸시가 노출
override func didReceive(_ request: UNNotificationRequest, withContentHandler contentHandler: @escaping (UNNotificationContent) -> Void) {
self.contentHandler = contentHandler
bestAttemptContent = (request.content.mutableCopy() as? UNMutableNotificationContent)
guard let bestAttemptContent else { return }
bestAttemptContent.title = "변경 " + request.content.title
bestAttemptContent.subtitle = "변경 " + request.content.subtitle
contentHandler(bestAttemptContent)
}
테스트
- 푸시 페이로드 준비
- NotificationServiceExtension의 페이로드는 "mutable-content": 1 가 필요
- 주의) Silent Push는 "content-available": 1 임을 주의
{
"aps" : {
"mutable-content": 1,
"alert" : {
"title" : "iOS 앱 개발 알아가기",
"subtitle" : "jake 서브 타이틀",
"body" : "바디"
},
"sound":"default"
}
}
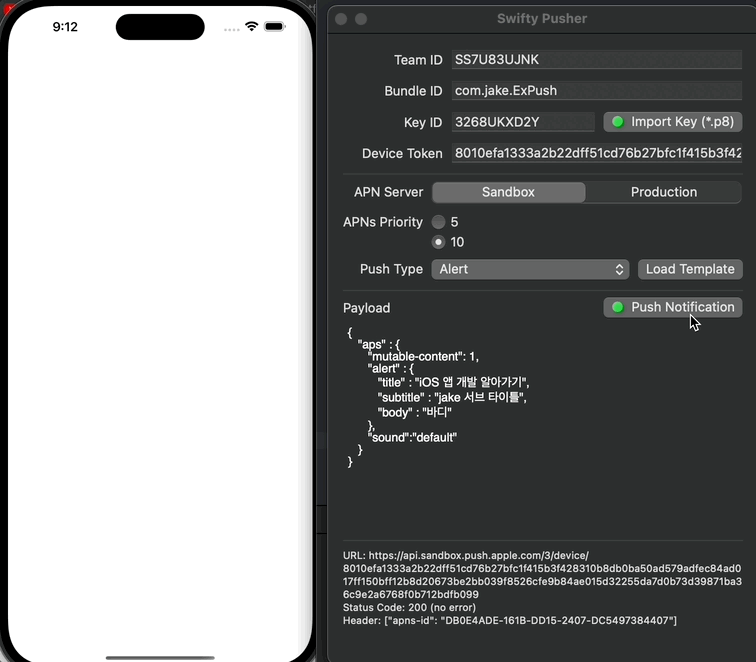
* 번외) 푸시 페이로드는 4KB로 제한되어, 이미지를 전달할때 url로 전달하면 앱에서 Notification Service Extension을 사용하여 푸시에 이미지를 다운받아서 푸시에 넣을 수 있는데, 방법은 이 포스팅 글 참고
* 전체 코드: https://github.com/JK0369/ExPushTest
* 참고
'iOS 응용 (swift)' 카테고리의 다른 글
jake-kim님의
글이 좋았다면 응원을 보내주세요!